记录输入的数据到文件 软件实现建立一个文件夹,在此文件夹中建立一个log记录文件(也是软件实现)。 键盘随机输入一个数,通过子线程把这个数记录到log文件里面去。 同时记录输入数据的时间,并且每次输入的数据不能覆盖前面输入的数据。 编译进程需要添加参数-lpthread
时间: 2023-12-03 08:43:17 浏览: 105
以下是一个简单的实现,使用了C语言的文件操作和线程库pthread:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <pthread.h>
#define MAX_INPUT_LEN 100
char log_file_path[100] = "./log/log.txt"; // 日志文件路径
// 获取当前时间字符串
void get_time_str(char *buf, int buf_len) {
time_t now;
struct tm *tm_now;
time(&now);
tm_now = localtime(&now);
strftime(buf, buf_len, "%Y-%m-%d %H:%M:%S", tm_now);
}
// 写日志
void write_log(const char *msg) {
FILE *fp = fopen(log_file_path, "a");
if (fp == NULL) {
printf("Failed to open log file!\n");
return;
}
char time_str[20];
get_time_str(time_str, sizeof(time_str));
fprintf(fp, "[%s] %s\n", time_str, msg);
fclose(fp);
}
// 线程函数,记录输入数据到日志文件
void *write_input_to_log(void *arg) {
char *input_data = (char *)arg;
write_log(input_data);
free(input_data); // 记得释放内存
pthread_exit(NULL);
}
int main() {
if (mkdir("./log", 0777) != 0 && errno != EEXIST) { // 创建日志文件夹
printf("Failed to create log directory!\n");
return 1;
}
while (1) {
char input[MAX_INPUT_LEN];
printf("Please input a number: ");
fgets(input, MAX_INPUT_LEN, stdin);
input[strlen(input)-1] = '\0'; // 去掉输入字符串的换行符
if (strcmp(input, "exit") == 0) { // 输入exit退出程序
break;
}
char *input_data = malloc(strlen(input)+20); // 分配内存,用于存储输入数据和时间字符串
if (input_data == NULL) {
printf("Failed to allocate memory!\n");
return 1;
}
snprintf(input_data, strlen(input)+20, "%s %s", input, "");
pthread_t tid;
if (pthread_create(&tid, NULL, write_input_to_log, (void *)input_data) != 0) { // 创建子线程写入日志文件
printf("Failed to create thread!\n");
free(input_data);
return 1;
}
}
return 0;
}
```
在编译时需要添加-lpthread参数,例如:
```
gcc -o main main.c -lpthread
```
当用户输入一个数字时,程序会创建一个子线程,把这个数字记录到日志文件里面去。日志文件记录了每次输入的数字和输入时间。程序可以通过输入exit来退出。每次输入的数字不会覆盖之前输入的数字,而是追加到日志文件的末尾。
阅读全文
相关推荐
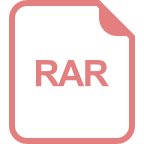
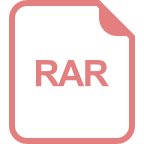
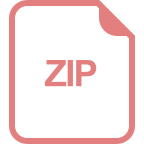
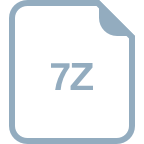
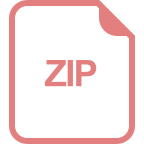
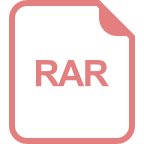
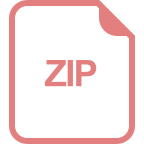
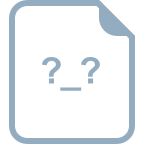
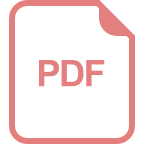
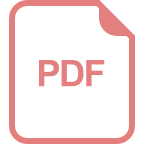
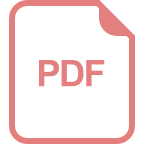
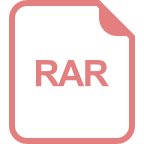
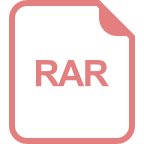
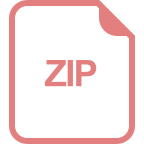
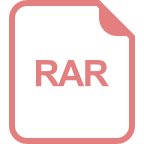