用stream流 map<string.Byte> 根据map的value排序
时间: 2024-02-27 07:58:51 浏览: 20
您可以使用C++ STL中的`std::map`和`std::vector`来实现这个功能。具体步骤如下:
1.定义一个`std::map<std::string, byte>`,并初始化它。
2.定义一个`std::vector<std::pair<std::string, byte>>`,并将map中的元素复制到vector中。
3.使用`std::sort`函数和一个lambda表达式来排序vector,lambda表达式应按照value值进行排序。
4.遍历排序后的vector,输出元素的key和value值。
示例代码如下:
```c++
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
using byte = unsigned char;
int main() {
std::map<std::string, byte> myMap = {{"hello", 3}, {"world", 2}, {"foo", 4}, {"bar", 1}};
std::vector<std::pair<std::string, byte>> myVector(myMap.begin(), myMap.end());
std::sort(myVector.begin(), myVector.end(), [](const std::pair<std::string, byte>& a, const std::pair<std::string, byte>& b) {
return a.second < b.second;
});
for (const auto& pair : myVector) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
输出结果为:
```
bar: 1
world: 2
hello: 3
foo: 4
```
相关推荐
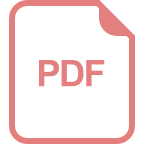
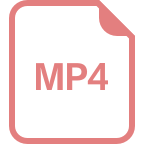














