TypeError: byte indices must be integers or slices, not tuple
时间: 2023-09-27 14:08:19 浏览: 310
这个错误通常是由于使用了元组作为字节索引的原因。在 Python 中,字节索引必须是整数或切片,不能是元组。
以下是一个例子:
```
s = b'hello'
print(s[0]) # 正确,输出 104
print(s[0, 1]) # 错误,抛出 TypeError
```
解决这个问题的方法是将元组转换为整数或切片。例如,将 `(0, 1)` 改为 `slice(0, 1)` :
```
s = b'hello'
print(s[slice(0, 1)]) # 正确,输出 b'h'
```
或者将 `(0, 1)` 改为单个整数,例如 `0` 或 `1`:
```
s = b'hello'
print(s[0]) # 正确,输出 104
print(s[1]) # 正确,输出 101
```
相关问题
TypeError: tuple indices must be integers or slices, not tuple
这个错误通常是由于尝试使用元组作为索引引起的。在 Python 中,元组的元素不能被更改,因此不能用元组作为索引。请确保您正在使用整数或切片作为索引。以下是一个示例:
```
my_tuple = ('a', 'b', 'c')
print(my_tuple[0]) # 输出 'a'
print(my_tuple[1:]) # 输出 ('b', 'c')
# 错误示例
print(my_tuple[0,1]) # TypeError: tuple indices must be integers or slices, not tuple
```
在上面的错误示例中,我们试图使用元组 `(0, 1)` 作为索引,导致出现 `TypeError`。正确的方式是使用单个整数或切片作为索引。
TypeError: list indices must be integers or slices, not tuple
这个错误通常发生在尝试使用元组作为列表的索引时。列表的索引应该是整数或切片,而不是元组。
例如,以下代码会引发这个错误:
```python
my_list = [1, 2, 3]
index = (0, 1)
value = my_list[index]
```
应该将索引改为整数或切片,例如:
```python
my_list = [1, 2, 3]
index = 1
value = my_list[index]
```
或者如果你想获取多个元素,你可以使用切片:
```python
my_list = [1, 2, 3]
indices = (0, 1)
values = my_list[indices[0]:indices[1]]
```
请检查你的代码,找到尝试使用元组作为列表索引的地方,并将其修改为适当的整数或切片。
阅读全文
相关推荐
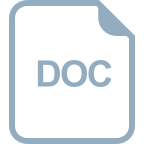
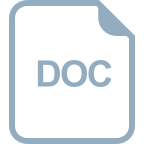
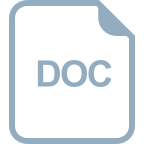



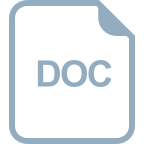
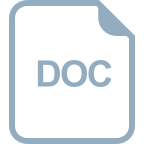
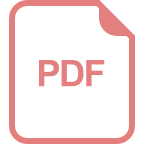
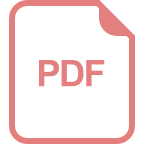
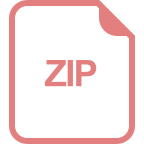