pygame我想通过添加按钮的方式,使用按钮打开一个用于说明操作规则的子页面,即可用字母“h”打开和关闭,也可使用鼠标点击打开或开闭,并将按钮位置设置在窗口位置
时间: 2024-03-22 08:40:13 浏览: 26
好的,你可以使用 Pygame 的 Sprite 类来创建按钮,然后在主循环中监听鼠标事件,当鼠标点击按钮时,打开或关闭子页面。具体步骤如下:
1. 导入 Pygame 模块:
```
import pygame
```
2. 初始化 Pygame:
```
pygame.init()
```
3. 定义颜色常量:
```
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
```
4. 创建按钮类:
```
class Button(pygame.sprite.Sprite):
def __init__(self, x, y, w, h, color, text):
super().__init__()
self.image = pygame.Surface((w, h))
self.image.fill(color)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
font = pygame.font.SysFont(None, 20)
text_surface = font.render(text, True, WHITE)
text_rect = text_surface.get_rect(center=(w/2, h/2))
self.image.blit(text_surface, text_rect)
```
5. 创建子页面类:
```
class SubPage(pygame.sprite.Sprite):
def __init__(self, x, y, w, h, color):
super().__init__()
self.image = pygame.Surface((w, h))
self.image.fill(color)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
```
6. 创建 Pygame 窗口:
```
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("My Game")
```
7. 创建按钮对象和子页面对象:
```
button = Button(10, 10, 100, 50, WHITE, "Help")
subpage = SubPage(150, 150, 500, 300, BLACK)
```
8. 创建精灵组,将按钮和子页面添加到精灵组:
```
all_sprites = pygame.sprite.Group()
all_sprites.add(button, subpage)
```
9. 在主循环中监听鼠标事件,当鼠标点击按钮时,打开或关闭子页面:
```
show_subpage = False
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_h:
show_subpage = not show_subpage
elif event.type == pygame.MOUSEBUTTONDOWN:
if button.rect.collidepoint(event.pos):
show_subpage = not show_subpage
screen.fill(BLACK)
all_sprites.draw(screen)
if show_subpage:
screen.blit(subpage.image, subpage.rect)
pygame.display.flip()
```
这样就可以创建一个带有按钮的 Pygame 窗口,并通过按钮打开或关闭一个子页面了。
相关推荐
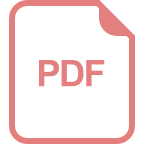













