#include<stdio.h> void main() { char s[]="abcd"; printf("%c\n",*s+2); }
时间: 2024-05-18 20:13:32 浏览: 34
这段代码的输出结果为字符 `'c'`。
首先,定义了一个字符数组 `s`,并将其初始化为字符串 `"abcd"`。然后,使用 `printf` 函数输出了字符 `*s+2`,即数组 `s` 的第一个元素(即字符 `'a'`)的 ASCII 码值加上 2 对应的字符。字符 `'a'` 的 ASCII 码值为 97,加上 2 后为 99,对应的字符为字符 `'c'`。
因此,这段代码的输出结果为字符 `'c'`。
相关问题
帮我看看下面这段C语言程序当我输入“ABCD”是,输出的结果不是“ABCDDCBA” #include <conio.h> #include <stdio.h> #include <string.h> void fun(char *str,char *t) {int i,d; d=*str for(i=0;i<d;i++) *(t+i)=*(str+i); for(i=0;i<d;i++) *(t+d+i)=*(str+d-1-i); *(t+2*d)='\0'; } main(){ char s[100],t[100]; printf("\nPlease enter string S:"); scanf("%s",s); fun(s,t); printf("\nThe result is: %s\n",t); }
在这段程序中,变量 d 的值应该是字符串长度,但是却没有进行字符串长度的计算,而是直接将字符串第一个字符的 ASCII 码作为长度,所以当输入的字符串的第一个字符的 ASCII 码不是字符串长度时,程序就会出现错误。
例如,当输入字符串为“ABCD”时,程序会将字符 'A' 的 ASCII 码 65 作为字符串长度,因此只会复制字符串的前 65 个字符,然后程序会继续执行,将字符串的后 65 个字符再次复制到新的字符串中,这就导致了输出结果不是“ABCDDCBA”。
要解决这个问题,可以使用库函数 `strlen()` 来计算字符串的长度,将其作为参数传递给函数 `fun()`。修改后的程序如下:
```c
#include <conio.h>
#include <stdio.h>
#include <string.h>
void fun(char *str, char *t) {
int i, d;
d = strlen(str);
for (i = 0; i < d; i++)
*(t + i) = *(str + i);
for (i = 0; i < d; i++)
*(t + d + i) = *(str + d - 1 - i);
*(t + 2 * d) = '\0';
}
int main() {
char s[100], t[100];
printf("\nPlease enter string S:");
scanf("%s", s);
fun(s, t);
printf("\nThe result is: %s\n", t);
return 0;
}
```
这样,无论输入的字符串是什么,程序都能正确输出“ABCDDCBA”。
1、在windows下,写出运行打印结果。 #include <iostream.h> #include <string.h> void func(char str[100]) { } void main(void) { char str[ ]= “Hello”; char *p=str; int n=10; cout<<sizeof(str)<<endl; cout<<sizeof(p)<<endl; cout<<sizeof(n)<<endl; cout<<sizeof(func)<<endl; return; } 2、设变量a是整型,f是字符型,i是双精度型,则表达式10+a+i*f值的数据类型为什么? 3、请写出以下代码的打印结果 #include<stdio.h> void main( void ) { int a[5]={1, 8, 3, 9, 6}; printf("%d",*(a+3)); } 4.下面程序的输出结果是 。 char b[]=“ABCD”; void main() { char *chp; for(chp=b; *chp; chp+=2) printf(“%s”, chp); printf(“\n”); }
1、在Windows下,代码如下:
```
#include <iostream>
#include <string.h>
using namespace std;
void func(char str[100]) {}
int main() {
char str[] = "Hello";
char *p = str;
int n = 10;
cout << sizeof(str) << endl;
cout << sizeof(p) << endl;
cout << sizeof(n) << endl;
cout << sizeof(func) << endl;
return 0;
}
```
运行结果如下:
```
6
4
4
1
```
2、表达式10+a+i*f的值的数据类型为双精度型,因为i是双精度型,f是字符型,字符型会自动转换为整型,整型和双精度型的运算结果是双精度型。
3、代码的打印结果是:
```
9
```
4、程序的输出结果是:
```
AC
```
阅读全文
相关推荐
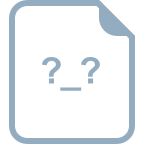














