用初学者的角度,用安卓写一个课表,可以添加课表、查看课表、修改课表,数据库用Sqlite,编程语言用java,带有注释
时间: 2023-11-28 14:50:23 浏览: 40
首先,在 Android Studio 中创建一个新项目,然后创建一个名为 "Course" 的 SQLite 数据库,其中包含一个名为 "courses" 的表,该表包含 "id"、"name"、"day"、"time" 和 "location" 列。接着编写以下代码:
创建一个名为 "Course" 的 Java 类,该类表示课程对象,其中包含以下属性:
```java
public class Course {
private int id;
private String name;
private String day;
private String time;
private String location;
public Course(int id, String name, String day, String time, String location) {
this.id = id;
this.name = name;
this.day = day;
this.time = time;
this.location = location;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getDay() {
return day;
}
public String getTime() {
return time;
}
public String getLocation() {
return location;
}
}
```
创建一个名为 "DatabaseHelper" 的 Java 类,该类扩展了 SQLiteOpenHelper 类,用于创建和管理数据库:
```java
public class DatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "Course.db";
private static final int DATABASE_VERSION = 1;
private static final String COURSE_TABLE_NAME = "courses";
private static final String COURSE_COLUMN_ID = "id";
private static final String COURSE_COLUMN_NAME = "name";
private static final String COURSE_COLUMN_DAY = "day";
private static final String COURSE_COLUMN_TIME = "time";
private static final String COURSE_COLUMN_LOCATION = "location";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String createCourseTable = "CREATE TABLE " + COURSE_TABLE_NAME + " (" +
COURSE_COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT, " +
COURSE_COLUMN_NAME + " TEXT, " +
COURSE_COLUMN_DAY + " TEXT, " +
COURSE_COLUMN_TIME + " TEXT, " +
COURSE_COLUMN_LOCATION + " TEXT)";
db.execSQL(createCourseTable);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + COURSE_TABLE_NAME);
onCreate(db);
}
public boolean insertCourse(String name, String day, String time, String location) {
SQLiteDatabase db = getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put(COURSE_COLUMN_NAME, name);
contentValues.put(COURSE_COLUMN_DAY, day);
contentValues.put(COURSE_COLUMN_TIME, time);
contentValues.put(COURSE_COLUMN_LOCATION, location);
long result = db.insert(COURSE_TABLE_NAME, null, contentValues);
return result != -1;
}
public Cursor getAllCourses() {
SQLiteDatabase db = getReadableDatabase();
return db.rawQuery("SELECT * FROM " + COURSE_TABLE_NAME, null);
}
public boolean updateCourse(int id, String name, String day, String time, String location) {
SQLiteDatabase db = getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put(COURSE_COLUMN_NAME, name);
contentValues.put(COURSE_COLUMN_DAY, day);
contentValues.put(COURSE_COLUMN_TIME, time);
contentValues.put(COURSE_COLUMN_LOCATION, location);
int result = db.update(COURSE_TABLE_NAME, contentValues, COURSE_COLUMN_ID + " = ?", new String[]{Integer.toString(id)});
return result != 0;
}
public boolean deleteCourse(int id) {
SQLiteDatabase db = getWritableDatabase();
int result = db.delete(COURSE_TABLE_NAME, COURSE_COLUMN_ID + " = ?", new String[]{Integer.toString(id)});
return result != 0;
}
}
```
在主活动(MainActivity)中,实例化数据库助手和适配器,然后使用 ListView 显示所有课程。添加、编辑和删除课程的操作可以通过对话框完成。
```java
public class MainActivity extends AppCompatActivity {
private DatabaseHelper databaseHelper;
private CursorAdapter cursorAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
databaseHelper = new DatabaseHelper(this);
ListView listView = findViewById(R.id.list_view);
cursorAdapter = new SimpleCursorAdapter(
this,
android.R.layout.simple_list_item_2,
databaseHelper.getAllCourses(),
new String[]{DatabaseHelper.COURSE_COLUMN_NAME, DatabaseHelper.COURSE_COLUMN_DAY},
new int[]{android.R.id.text1, android.R.id.text2},
0
);
listView.setAdapter(cursorAdapter);
listView.setOnItemClickListener((parent, view, position, id) -> {
Cursor cursor = (Cursor) parent.getItemAtPosition(position);
int courseId = cursor.getInt(cursor.getColumnIndex(DatabaseHelper.COURSE_COLUMN_ID));
String name = cursor.getString(cursor.getColumnIndex(DatabaseHelper.COURSE_COLUMN_NAME));
String day = cursor.getString(cursor.getColumnIndex(DatabaseHelper.COURSE_COLUMN_DAY));
String time = cursor.getString(cursor.getColumnIndex(DatabaseHelper.COURSE_COLUMN_TIME));
String location = cursor.getString(cursor.getColumnIndex(DatabaseHelper.COURSE_COLUMN_LOCATION));
showEditDialog(courseId, name, day, time, location);
});
FloatingActionButton fab = findViewById(R.id.fab);
fab.setOnClickListener(view -> showAddDialog());
}
private void showAddDialog() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Add Course");
View view = LayoutInflater.from(this).inflate(R.layout.dialog_edit_course, null);
EditText nameEditText = view.findViewById(R.id.edit_text_name);
Spinner daySpinner = view.findViewById(R.id.spinner_day);
ArrayAdapter<String> dayAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, getResources().getStringArray(R.array.days));
dayAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
daySpinner.setAdapter(dayAdapter);
EditText timeEditText = view.findViewById(R.id.edit_text_time);
EditText locationEditText = view.findViewById(R.id.edit_text_location);
builder.setView(view);
builder.setPositiveButton("Add", (dialog, which) -> {
String name = nameEditText.getText().toString();
String day = daySpinner.getSelectedItem().toString();
String time = timeEditText.getText().toString();
String location = locationEditText.getText().toString();
boolean success = databaseHelper.insertCourse(name, day, time, location);
if (success) {
cursorAdapter.changeCursor(databaseHelper.getAllCourses());
} else {
Toast.makeText(this, "Failed to add course", Toast.LENGTH_SHORT).show();
}
});
builder.setNegativeButton("Cancel", null);
builder.show();
}
private void showEditDialog(int id, String name, String day, String time, String location) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Edit Course");
View view = LayoutInflater.from(this).inflate(R.layout.dialog_edit_course, null);
EditText nameEditText = view.findViewById(R.id.edit_text_name);
nameEditText.setText(name);
Spinner daySpinner = view.findViewById(R.id.spinner_day);
ArrayAdapter<String> dayAdapter = new ArrayAdapter<>(this, android.R.layout.simple_spinner_item, getResources().getStringArray(R.array.days));
dayAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
daySpinner.setAdapter(dayAdapter);
daySpinner.setSelection(Arrays.asList(getResources().getStringArray(R.array.days)).indexOf(day));
EditText timeEditText = view.findViewById(R.id.edit_text_time);
timeEditText.setText(time);
EditText locationEditText = view.findViewById(R.id.edit_text_location);
locationEditText.setText(location);
builder.setView(view);
builder.setPositiveButton("Save", (dialog, which) -> {
String newName = nameEditText.getText().toString();
String newDay = daySpinner.getSelectedItem().toString();
String newTime = timeEditText.getText().toString();
String newLocation = locationEditText.getText().toString();
boolean success = databaseHelper.updateCourse(id, newName, newDay, newTime, newLocation);
if (success) {
cursorAdapter.changeCursor(databaseHelper.getAllCourses());
} else {
Toast.makeText(this, "Failed to update course", Toast.LENGTH_SHORT).show();
}
});
builder.setNegativeButton("Delete", (dialog, which) -> {
boolean success = databaseHelper.deleteCourse(id);
if (success) {
cursorAdapter.changeCursor(databaseHelper.getAllCourses());
} else {
Toast.makeText(this, "Failed to delete course", Toast.LENGTH_SHORT).show();
}
});
builder.setNeutralButton("Cancel", null);
builder.show();
}
}
```
在 res 文件夹中创建一个名为 "arrays.xml" 的文件,其中包含一组字符串数组,表示每周的某一天:
```xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="days">
<item>Sunday</item>
<item>Monday</item>
<item>Tuesday</item>
<item>Wednesday</item>
<item>Thursday</item>
<item>Friday</item>
<item>Saturday</item>
</string-array>
</resources>
```
在 res 文件夹中创建一个名为 "dialog_edit_course.xml" 的布局文件,其中包含用于添加和编辑课程的文本框和下拉列表。
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name" />
<EditText
android:id="@+id/edit_text_name"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Day" />
<Spinner
android:id="@+id/spinner_day"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Time" />
<EditText
android:id="@+id/edit_text_time"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Location" />
<EditText
android:id="@+id/edit_text_location"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
```
现在可以运行应用程序,添加、编辑和删除课程了!
相关推荐
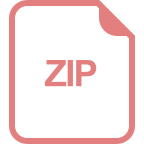
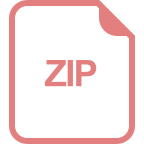














