使用JavaScript制作新年倒计时和轮播图
时间: 2024-10-17 13:11:52 浏览: 90
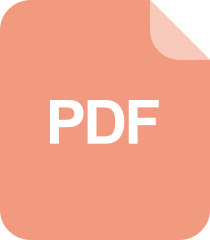
JavaScript实现新年倒计时效果
在JavaScript中制作新年倒计时和轮播图通常涉及到前端交互和定时功能。以下是简要步骤:
1. **新年倒计时**:
- 首先,获取当前年份和目标年份(例如新的一年),然后计算剩余的时间差。
- 使用`setInterval()`函数每秒更新时间,并减去一年的总秒数(365天 * 24小时 * 60分钟 * 60秒)。
- 当时间差减少到零时,显示倒计时结束的消息或者开始新的年份倒计时。
```javascript
const targetYear = new Date().getFullYear() + 1;
let countdown = 0;
function updateCountdown() {
countdown--;
if (countdown <= 0) {
// 新年到来,切换至新年的倒计时
countdown = newDate(targetYear).getDate() * 24 * 60 * 60 - Date.now();
}
document.getElementById("new-year-countdown").innerText = "距离新年还有" + countdown + "秒";
}
setInterval(updateCountdown, 1000);
```
2. **轮播图**:
- 创建一个包含图片的容器和一些指示器按钮。
- 设置初始图像显示,同时隐藏后续图片和按钮。
- 使用`setTimeout()`或`requestAnimationFrame()`创建动画效果,比如每隔一定时间改变当前显示的图片并移动指示器。
- 添加事件监听器,以便用户点击按钮时跳转到相应的位置。
```javascript
let currentIndex = 0;
const images = document.querySelectorAll('.carousel-image');
const indicators = document.querySelectorAll('.indicator-button');
function slideShow() {
images[currentIndex].style.display = 'block';
indicators[currentIndex].classList.add('active');
setTimeout(() => {
currentIndex = (currentIndex + 1) % images.length; // 循环处理
images[currentIndex].style.display = 'none'; // 隐藏当前显示的图片
slideShow();
}, 3000); // 每隔3秒切换一张图片
}
slideShow(); // 开始轮播
indicators.forEach((button, index) => {
button.addEventListener('click', () => {
currentIndex = index;
images[currentIndex].style.display = 'block';
indicators[currentIndex].classList.add('active');
setTimeout(() => {
slideShow();
}, 0);
});
});
```
阅读全文
相关推荐
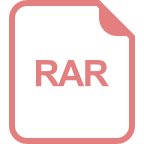
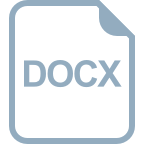
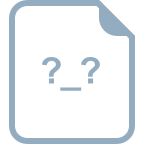
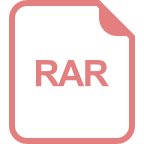
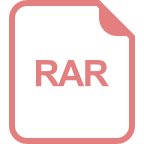
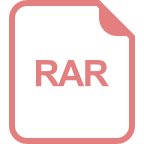
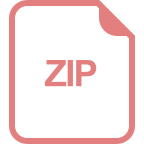
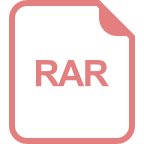
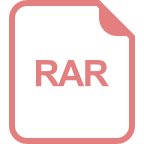
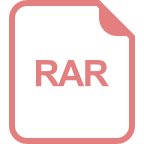
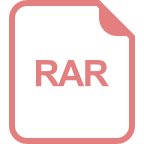
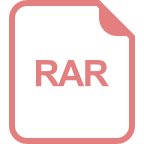
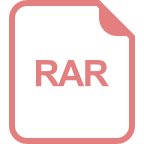
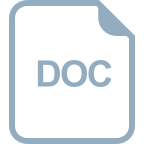
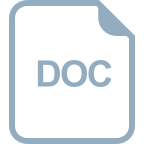
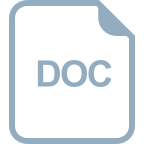
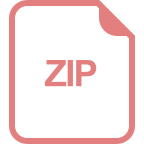
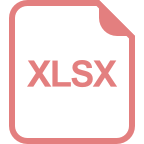