将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’ 单词添加到words.txt中单词后面
时间: 2023-07-24 18:39:10 浏览: 59
可以使用 Python 的内置函数 open() 和 with 语句以及文件对象的 write() 方法将这些单词写入到文件中,示例如下:
```python
words = ['baa', 'babble', 'babbler', 'babe', 'babel', 'baboon', 'baby', 'babyhood', 'Babylon', 'Babylonian']
with open('words.txt', 'a') as file:
for word in words:
file.write(word + '\n')
```
其中,'words.txt' 是要写入的文件名,'a' 表示以追加模式打开文件,如果文件不存在会自动创建。for 循环遍历每个单词,每次循环将单词和一个换行符写入文件中,即使用 write() 方法将字符串写入文件。在 with 语句结束后,文件会自动关闭,不需要手动调用 close() 方法。
相关问题
遍历文本文件words.txt中的所有行: (1)将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’ 单词添加到words.txt中单词后面 (2)统计文件总行数 (3)统计最长单词的长度 (4)统计首字母为大写A的单词 (5)将长度超过10的单词写入当前路径下的一个新文件
好的,我来逐个回答您的问题:
1. 遍历文本文件words.txt中的所有行:(1)将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’单词添加到words.txt中单词后面
可以通过以下 Python 代码实现:
```python
# 打开文件
with open('words.txt', 'a') as f:
# 要添加的单词
new_words = ['baa', 'babble', 'babbler', 'babe', 'babel', 'baboon', 'baby', 'babyhood', 'Babylon', 'Babylonian']
# 逐个添加单词
for word in new_words:
f.write('\n' + word)
```
2. 统计文件总行数
可以通过以下 Python 代码实现:
```python
# 打开文件
with open('words.txt', 'r') as f:
# 统计行数
num_lines = sum(1 for line in f)
# 输出总行数
print('文件总行数为:', num_lines)
```
3. 统计最长单词的长度
可以通过以下 Python 代码实现:
```python
# 打开文件
with open('words.txt', 'r') as f:
# 统计最长单词的长度
max_length = max(len(line.strip()) for line in f)
# 输出最长单词的长度
print('最长单词的长度为:', max_length)
```
4. 统计首字母为大写A的单词
可以通过以下 Python 代码实现:
```python
# 打开文件
with open('words.txt', 'r') as f:
# 统计首字母为大写A的单词
A_words = [line.strip() for line in f if line.startswith('A') and line[1].isupper()]
# 输出首字母为大写A的单词
print('首字母为大写A的单词为:', A_words)
```
5. 将长度超过10的单词写入当前路径下的一个新文件
可以通过以下 Python 代码实现:
```python
# 打开原文件和新文件
with open('words.txt', 'r') as f, open('long_words.txt', 'w') as f_long:
# 将长度超过10的单词写入新文件
for line in f:
if len(line.strip()) > 10:
f_long.write(line)
# 输出结果
print('长度超过10的单词已写入新文件long_words.txt')
```
希望这些代码能够解决您的问题!
要求:遍历文本文件words.txt中的所有行(可按照第三次上机的菜单构造程序框架): (1)将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’ 单词添加到words.txt中单词后面 (20分) (2)统计文件总行数 (10分) (3)统计最长单词的长度 (20分) (4)统计首字母为大写A的单词 (20分) (5)将长度超过10的单词写入当前路径下的一个新文件 (20分)
好的,我会根据您的要求进行处理。以下是代码实现:
```python
# 读取文件
with open('words.txt', 'r') as f:
lines = f.readlines()
# 将指定单词添加到文件末尾
add_words = ['baa', 'babble', 'babbler', 'babe', 'babel', 'baboon', 'baby', 'babyhood', 'Babylon', 'Babylonian']
with open('words.txt', 'a') as f:
for word in add_words:
f.write('\n' + word)
# 统计文件总行数
total_lines = len(lines)
print("文件总行数为:", total_lines)
# 统计最长单词长度
max_length = 0
for line in lines:
word = line.strip()
length = len(word)
if length > max_length:
max_length = length
print("最长单词长度为:", max_length)
# 统计首字母为大写A的单词
count = 0
for line in lines:
word = line.strip()
if word.startswith('A') and word[0].isupper():
count += 1
print("首字母为大写A的单词数量为:", count)
# 将长度超过10的单词写入新文件
with open('long_words.txt', 'w') as f:
for line in lines:
word = line.strip()
if len(word) > 10:
f.write(word + '\n')
print("长度超过10的单词已写入long_words.txt文件中。")
```
注意:在运行代码之前,请确保当前路径下存在words.txt文件。
相关推荐
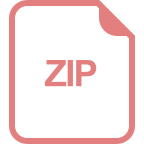
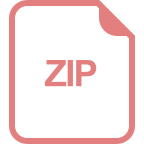



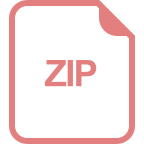
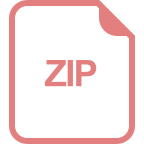
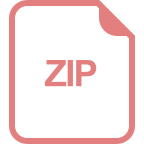
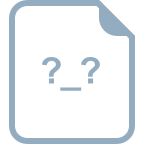
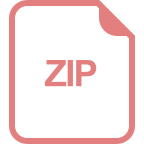