C++求点集最小包围球
时间: 2024-11-09 15:23:32 浏览: 30
在C++中,寻找点集的最小包围球,也称为最小外接球,可以使用多种方法。常见的有直接法、间接法以及一些优化过的算法,比如Weiszfeld算法或Welzl's Algorithm等。这里以一种简单的直接法为例:
**直接法:**
首先,你需要遍历所有的点对,计算它们之间的最大距离,这个最大距离将是最小包围球直径的一半。然后,你可以从任意一点出发,通过逐步减小直径的方式尝试找到球心。每一步都检查新直径下的所有点是否都在球内。这是一个暴力搜索,适用于较小规模的点集。
```cpp
#include <iostream>
#include <vector>
#include <cmath>
struct Point {
double x, y;
};
double distance(const Point& a, const Point& b) {
return std::sqrt(std::pow(a.x - b.x, 2) + std::pow(a.y - b.y, 2));
}
Point find_sphere_center(const std::vector<Point>& points) {
double max_distance = 0;
for (const auto& point1 : points) {
for (const auto& point2 : points) {
max_distance = std::max(max_distance, distance(point1, point2));
}
}
double dx = 0, dy = 0;
while (true) {
Point center = {dx, dy};
bool in_sphere = true;
for (const auto& point : points) {
if (distance(center, point) > max_distance + std::sqrt(std::pow(dx, 2) + std::pow(dy, 2))) {
in_sphere = false;
break;
}
}
if (in_sphere) break;
double step = max_distance / 2;
if (step <= std::numeric_limits<double>::epsilon()) break; // 足够精确了
if (center.x > 0 && distance({center.x - step, dy}, points[0]) > max_distance) {
dx -= step;
} else if (center.x < points.back().x && distance({center.x + step, dy}, points.back()) > max_distance) {
dx += step;
} else if (center.y > 0 && distance({dx, center.y - step}, points[0]) > max_distance) {
dy -= step;
} else if (center.y < points.back().y && distance({dx, center.y + step}, points.back()) > max_distance) {
dy += step;
}
}
return center;
}
int main() {
std::vector<Point> points = {{0, 0}, {1, 1}, {2, 2}};
Point center = find_sphere_center(points);
std::cout << "最小包围球中心: (" << center.x << ", " << center.y << ")\n";
return 0;
}
```
阅读全文
相关推荐
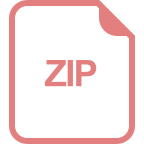
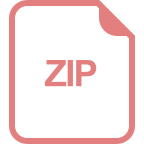
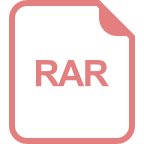






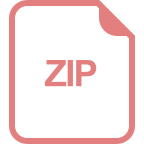
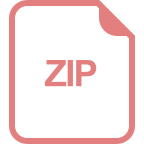
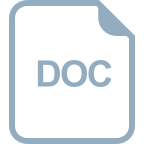
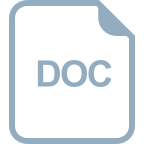
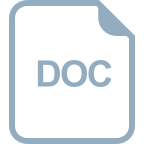




