构造一个单链表,该链表由三个结点构成,结点中的数据部分包括:学号、成绩。现要把三个结点的信息输出。用
时间: 2024-03-17 20:46:40 浏览: 59
C语言实现如下:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表结点
struct ListNode {
int num; // 学号
float score; // 成绩
struct ListNode *next; // 下一个结点的指针
};
int main() {
// 创建链表结点
struct ListNode *node1 = (struct ListNode*)malloc(sizeof(struct ListNode));
node1->num = 1001;
node1->score = 90.5;
struct ListNode *node2 = (struct ListNode*)malloc(sizeof(struct ListNode));
node2->num = 1002;
node2->score = 85.5;
struct ListNode *node3 = (struct ListNode*)malloc(sizeof(struct ListNode));
node3->num = 1003;
node3->score = 92.0;
// 将结点连接成链表
node1->next = node2;
node2->next = node3;
node3->next = NULL;
// 遍历链表并输出每个结点的信息
struct ListNode *p = node1;
while (p != NULL) {
printf("学号:%d,成绩:%.1f\n", p->num, p->score);
p = p->next;
}
// 释放内存
free(node1);
free(node2);
free(node3);
return 0;
}
```
输出结果为:
```
学号:1001,成绩:90.5
学号:1002,成绩:85.5
学号:1003,成绩:92.0
```
阅读全文
相关推荐
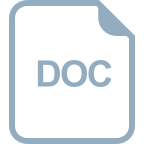
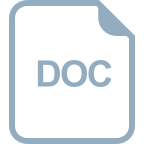
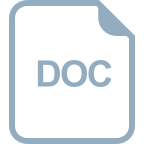
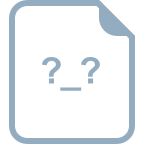














