python实现电梯算法
时间: 2023-11-25 14:51:23 浏览: 227
以下是Python模拟简单电梯调度算法的示例代码,其中使用了Python线程、队列、时间延迟等相关操作技巧:
```python
import threading
import time
from queue import Queue
class Elevator:
def __init__(self, num_floors):
self.num_floors = num_floors
self.current_floor = 1
self.direction = 1 # 1 for up, -1 for down
self.queue = Queue()
self.is_running = False
def add_to_queue(self, floor):
if floor == self.current_floor:
print(f"Elevator is already on floor {floor}")
return
if floor < 1 or floor > self.num_floors:
print(f"Invalid floor {floor}")
return
self.queue.put(floor)
def start(self):
if self.is_running:
print("Elevator is already running")
return
self.is_running = True
t = threading.Thread(target=self.run)
t.start()
def stop(self):
self.is_running = False
def run(self):
while not self.queue.empty():
next_floor = self.queue.get()
if next_floor > self.current_floor:
self.direction = 1
else:
self.direction = -1
while self.current_floor != next_floor:
self.current_floor += self.direction
print(f"Elevator is on floor {self.current_floor}")
time.sleep(1)
print(f"Elevator has arrived at floor {self.current_floor}")
self.is_running = False
# 示例代码
elevator = Elevator(10)
elevator.add_to_queue(5)
elevator.add_to_queue(3)
elevator.add_to_queue(8)
elevator.start()
time.sleep(10)
elevator.stop()
```
阅读全文
相关推荐
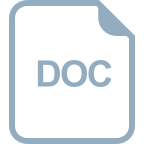
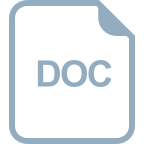
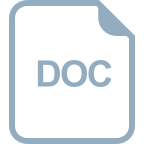


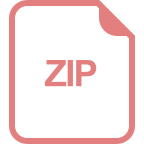
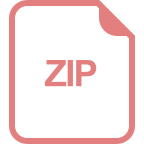
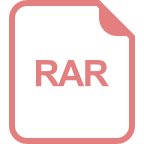
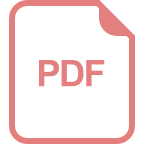
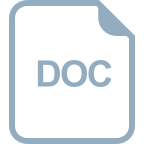
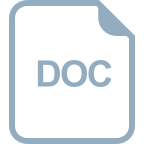



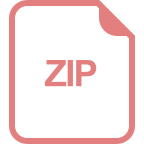

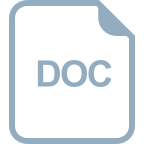
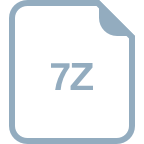