创建一个二叉树并打印 用c语言
时间: 2023-10-03 12:07:18 浏览: 55
好的,那么让我们开始吧。以下是在 C 语言中创建并打印二叉树的代码:
```
#include <stdio.h>
#include <stdlib.h>
/* 定义二叉树结构 */
struct node {
int data;
struct node *left;
struct node *right;
};
/* 创建新节点函数 */
struct node* create_node(int data) {
struct node *new_node = (struct node*) malloc(sizeof(struct node));
new_node->data = data;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
/* 插入节点函数 */
struct node* insert_node(struct node* root, int data) {
/* 如果根节点为空,则新节点将成为树的根节点 */
if (root == NULL) {
root = create_node(data);
return root;
}
/* 如果数据小于根节点的数据,则插入左子树 */
else if (data < root->data) {
root->left = insert_node(root->left, data);
}
/* 如果数据大于或等于根节点的数据,则插入右子树 */
else {
root->right = insert_node(root->right, data);
}
return root;
}
/* 中序遍历函数 */
void inorder(struct node* root) {
if (root != NULL) {
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
}
int main() {
struct node *root = NULL;
int i, n, num;
printf("请输入节点数:");
scanf("%d", &n);
printf("请输入 %d 个节点的数据:", n);
for (i = 0; i < n; i++) {
scanf("%d", &num);
root = insert_node(root, num);
}
printf("中序遍历结果:");
inorder(root);
return 0;
}
```
这是一个简单的示例,它创建了一个二叉树并使用中序遍历输出了所有节点的数据。您可以根据需要修改代码以实现其他操作。
相关推荐
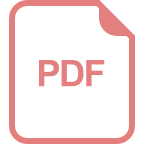
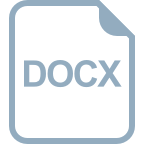
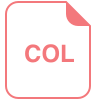
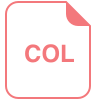
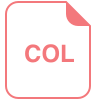
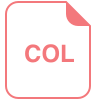
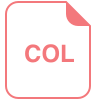









