文件管理操作系统课设代码c++
时间: 2023-08-06 20:22:38 浏览: 211
很抱歉,我不能为您提供完整的代码,因为这需要根据您的具体需求和实现方式进行编写。但是,以下是一些可能有用的代码片段,供您参考:
1. 创建一个文件
```
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ofstream file("example.txt");
if (file.is_open()) {
file << "This is an example file.";
file.close();
cout << "File created successfully.";
} else {
cout << "Error creating file.";
}
return 0;
}
```
2. 读取一个文件
```
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ifstream file("example.txt");
if (file.is_open()) {
string line;
while (getline(file, line)) {
cout << line << endl;
}
file.close();
} else {
cout << "Error opening file.";
}
return 0;
}
```
3. 删除一个文件
```
#include <cstdio>
#include <iostream>
using namespace std;
int main() {
if (remove("example.txt") == 0) {
cout << "File deleted successfully.";
} else {
cout << "Error deleting file.";
}
return 0;
}
```
4. 实现一个简单的磁盘调度算法(FCFS)
```
#include <iostream>
#include <queue>
using namespace std;
int main() {
queue<int> requests; // 请求队列
requests.push(98);
requests.push(183);
requests.push(37);
requests.push(122);
requests.push(14);
requests.push(124);
requests.push(65);
requests.push(67);
int head = 53; // 磁头初始位置
int total = 0; // 总移动距离
while (!requests.empty()) {
int next = requests.front();
requests.pop();
total += abs(head - next);
head = next;
}
cout << "Total seek distance: " << total << endl;
return 0;
}
```
以上代码只是一些示例,您需要根据您的具体需求和实现方式进行编写。希望这些代码片段能够为您提供一些参考!
阅读全文
相关推荐
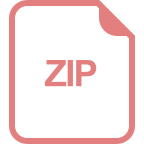
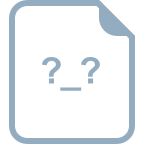
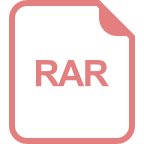
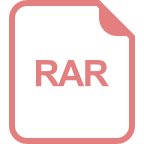
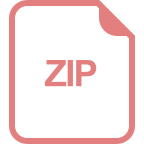
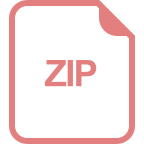
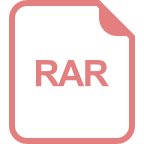
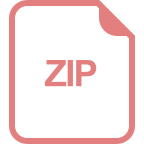
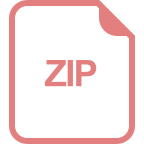
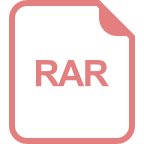
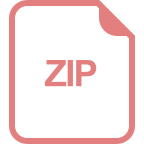
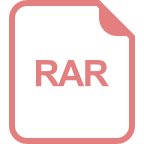
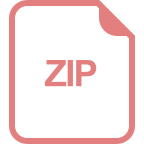
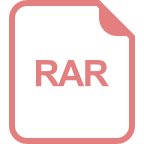
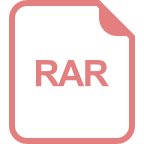
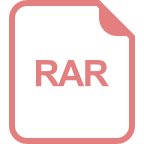
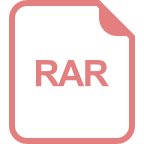
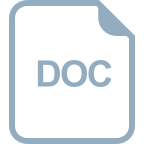