vue中实现验证码的功能
时间: 2023-05-29 08:03:42 浏览: 340
在Vue中实现验证码的功能,可以借助第三方库或自行实现。
1. 借助第三方库
可以使用第三方库vue-verify-code,该库提供了一个名为VerifyCode的组件,可以直接在Vue中使用。
安装:
```
npm install vue-verify-code --save
```
使用:
```html
<template>
<div>
<verify-code :options="options" @change="handleChange" />
<button @click="refresh">刷新验证码</button>
</div>
</template>
<script>
import VerifyCode from 'vue-verify-code'
export default {
components: {
VerifyCode
},
data () {
return {
options: {
len: 4,
width: 100,
height: 40
},
code: ''
}
},
methods: {
handleChange (code) {
this.code = code
},
refresh () {
this.$refs.verifyCode.refresh()
}
}
}
</script>
```
2. 自行实现
可以自行实现一个验证码组件,在组件中生成随机字符串,并将其显示为图片。用户输入验证码后,将用户输入的值与生成的随机字符串进行比较,判断是否匹配。
以下是一个简单的自行实现验证码组件的示例:
```html
<template>
<div class="captcha">
<canvas ref="canvas" width="100" height="40"></canvas>
<input type="text" v-model="value" @input="handleInput">
</div>
</template>
<script>
export default {
data () {
return {
value: '',
code: ''
}
},
mounted () {
this.generateCode()
},
methods: {
generateCode () {
const chars = 'ABCDEFGHJKMNPQRSTWXYZabcdefhijkmnprstwxyz2345678'
let code = ''
for (let i = 0; i < 4; i++) {
code += chars[Math.floor(Math.random() * chars.length)]
}
this.code = code
this.drawCode(code)
},
drawCode (code) {
const canvas = this.$refs.canvas
const ctx = canvas.getContext('2d')
ctx.clearRect(0, 0, canvas.width, canvas.height)
ctx.font = 'bold 30px Arial'
ctx.fillStyle = '#333'
ctx.textAlign = 'center'
ctx.textBaseline = 'middle'
ctx.fillText(code, canvas.width / 2, canvas.height / 2)
},
handleInput () {
this.$emit('input', this.value)
}
},
watch: {
value (val) {
if (val.length === 4 && val.toLowerCase() === this.code.toLowerCase()) {
this.$emit('success')
}
}
}
}
</script>
```
使用:
```html
<template>
<div>
<captcha @success="handleSuccess" v-model="captcha" />
<button @click="refresh">刷新验证码</button>
</div>
</template>
<script>
import Captcha from './Captcha.vue'
export default {
components: {
Captcha
},
data () {
return {
captcha: ''
}
},
methods: {
handleSuccess () {
alert('验证码正确')
},
refresh () {
this.$refs.captcha.generateCode()
this.captcha = ''
}
}
}
</script>
```
相关推荐
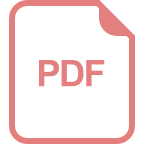
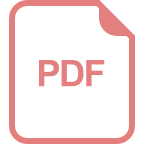














