ESP32-C3, esp-idf 4.3.5,用spi接口配置ch623读打印读取b卡的卡号
时间: 2024-02-22 11:55:08 浏览: 135
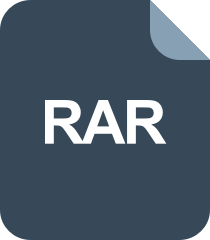
在ESP32-C3芯片上,展示如何使用ESP-IDF环境的API计算MD5值
好的,对于这个问题,你需要先安装 ESP-IDF 开发框架,然后根据你的硬件连接情况,配置 ESP32-C3 的 SPI 接口,并且在代码中使用 SPI 接口读取 CH623 芯片的卡号。
以下是一个简单的代码示例,可以帮助你开始编写读取卡号的代码:
```c
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/spi_master.h"
#define PIN_NUM_MISO 19
#define PIN_NUM_MOSI 23
#define PIN_NUM_CLK 18
#define PIN_NUM_CS 5
#define CMD_READ_CARD 0x30
spi_device_handle_t spi;
void app_main(void)
{
esp_err_t ret;
spi_bus_config_t buscfg={
.miso_io_num=PIN_NUM_MISO,
.mosi_io_num=PIN_NUM_MOSI,
.sclk_io_num=PIN_NUM_CLK,
.quadwp_io_num=-1,
.quadhd_io_num=-1,
};
spi_device_interface_config_t devcfg={
.clock_speed_hz=1*1000*1000, //Clock out at 1 MHz
.mode=0, //SPI mode 0
.spics_io_num=PIN_NUM_CS, //CS pin
.queue_size=7, //We want to be able to queue 7 transactions at a time
};
//Initialize the SPI bus
ret=spi_bus_initialize(HSPI_HOST, &buscfg, 1);
ESP_ERROR_CHECK(ret);
//Attach the CH623 to the SPI bus
ret=spi_bus_add_device(HSPI_HOST, &devcfg, &spi);
ESP_ERROR_CHECK(ret);
//Read the card number from CH623
uint8_t tx_data[1] = {CMD_READ_CARD};
uint8_t rx_data[4];
spi_transaction_t t={
.length=8, //Send 8 bits
.tx_buffer=tx_data, //The data is the cmd itself
.rx_buffer=rx_data, //Rx buffer
.user=(void*)0, //D/C needs to be set to 0
};
ret=spi_device_polling_transmit(spi, &t); //Transmit!
ESP_ERROR_CHECK(ret);
//Print the card number
printf("Card number: %02x%02x%02x%02x\n", rx_data[0], rx_data[1], rx_data[2], rx_data[3]);
//Free the SPI bus
spi_bus_remove_device(spi);
spi_bus_free(HSPI_HOST);
}
```
对于这个示例代码,你需要注意以下几点:
1. 根据你的硬件连接情况,修改代码中的 PIN_NUM_MISO、PIN_NUM_MOSI、PIN_NUM_CLK 和 PIN_NUM_CS 宏定义。
2. CH623 芯片的读取命令是 0x30,你需要在读取卡号之前,先向 CH623 发送这个命令。
3. CH623 读取的卡号为 4 字节,需要使用数组 rx_data 来存储读取到的数据。
4. 示例代码使用了轮询模式,如果你需要使用中断模式或 DMA 模式,需要修改相应的代码。
希望这个示例代码能够帮助到你,如果你有其他问题,可以随时问我。
阅读全文
相关推荐
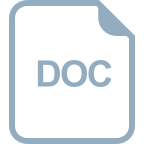
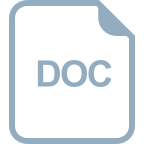






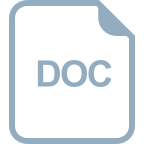
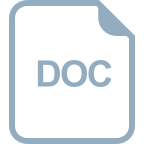
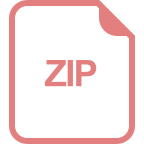
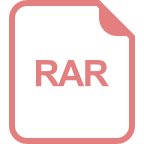
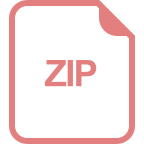
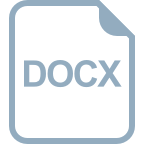
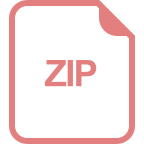