C语言中strlen,strcpy,strcat,strcmp分别举例
时间: 2023-03-23 21:01:23 浏览: 131
好的,我明白了。以下是C语言中字符串函数strlen、strcpy、strcat和strcmp的例子:
1. strlen函数返回字符串的长度,不包括末尾的空字符'\0'。例如:
```
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "hello world";
int len = strlen(str);
printf("Length of string '%s' is %d\n", str, len);
return 0;
}
```
输出:Length of string 'hello world' is 11
2. strcpy函数将一个字符串复制到另一个字符串中。例如:
```
#include <stdio.h>
#include <string.h>
int main() {
char src[] = "hello world";
char dest[20];
strcpy(dest, src);
printf("Source string: '%s'\n", src);
printf("Destination string: '%s'\n", dest);
return 0;
}
```
输出:Source string: 'hello world'
Destination string: 'hello world'
3. strcat函数将一个字符串连接到另一个字符串的末尾。例如:
```
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "hello";
char str2[] = " world";
strcat(str1, str2);
printf("Concatenated string: '%s'\n", str1);
return 0;
}
```
输出:Concatenated string: 'hello world'
4. strcmp函数比较两个字符串是否相等。例如:
```
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "hello";
char str2[] = "world";
int result = strcmp(str1, str2);
if (result == 0) {
printf("Strings are equal\n");
} else {
printf("Strings are not equal\n");
}
return 0;
}
```
输出:Strings are not equal
阅读全文
相关推荐
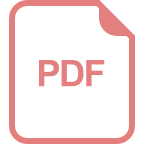
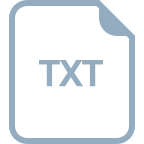
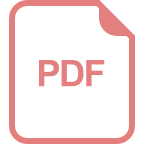















