qt QPushButton数组如何创建?
时间: 2024-03-14 19:46:32 浏览: 211
在 Qt 中,你可以使用数组来存储 QPushButton 对象。下面是创建 QPushButton 数组的示例代码:
```cpp
#include <QPushButton>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QPushButton* buttons[3]; // 创建一个包含3个 QPushButton 指针的数组
for(int i=0; i<3; i++)
{
buttons[i] = new QPushButton(QString("Button %1").arg(i+1)); // 创建 QPushButton 对象,并将指针存储到数组中
buttons[i]->show(); // 显示按钮
}
return app.exec();
}
```
在上面的代码中,我们创建了一个包含3个 QPushButton 指针的数组,然后使用 for 循环遍历数组,为每个指针创建一个 QPushButton 对象,并将其存储到数组中。最后,我们使用 show() 方法显示按钮。
需要注意的是,当使用 new 运算符创建 QPushButton 对象时,需要手动管理内存,即在不需要使用按钮时,需要使用 delete 运算符释放内存,以避免内存泄漏。
相关问题
qt QPushButton数组如何批量添加元素?
如果你想要在 Qt 中,使用 QPushButton 数组批量添加元素,可以使用类似 QVector 或 QList 的方式,可以使用数组初始化列表、循环等方法。下面是两种方式的示例:
1. 使用数组初始化列表
```cpp
QPushButton *buttons[] = {
new QPushButton("Button 1"),
new QPushButton("Button 2"),
new QPushButton("Button 3")
};
// 将按钮添加到布局
for (int i = 0; i < 3; i++) {
layout->addWidget(buttons[i]);
}
```
上面的代码中,先定义了一个 QPushButton 指针数组 buttons,并使用花括号将三个 QPushButton 对象作为数组元素进行初始化。然后使用循环将按钮添加到布局中。
2. 使用循环
```cpp
QPushButton *buttons[3];
// 创建按钮并添加到数组
for (int i = 0; i < 3; i++) {
buttons[i] = new QPushButton(QString("Button %1").arg(i+1));
}
// 将按钮添加到布局
for (int i = 0; i < 3; i++) {
layout->addWidget(buttons[i]);
}
```
上面的代码中,先定义了一个 QPushButton 指针数组 buttons,然后使用循环创建三个 QPushButton 对象并将其添加到数组中。最后使用循环将按钮添加到布局中。
无论是数组初始化列表还是循环,都可以批量添加 QPushButton 对象到数组中。
qt 如何获得QPushButton数组的元素个数?
如果你在代码中定义了一个 `QPushButton` 数组,你可以通过以下代码获取元素个数:
```c++
QPushButton *buttons[] = {button1, button2, button3, button4, button5};
int count = sizeof(buttons) / sizeof(QPushButton*);
```
这里假设你已经定义了一个 `QPushButton` 数组 `buttons`,包含了五个按钮对象 `button1`、`button2`、`button3`、`button4` 和 `button5`。在上面的代码中,我们使用了 `sizeof` 操作符来获取 `QPushButton*` 指针的大小,并用 `sizeof(buttons)` 来获取整个数组的大小。通过将这两个值相除,我们就可以得到 `buttons` 数组中元素的个数。
请注意,这种方法只适用于静态定义的数组。如果你在运行时动态创建了 `QPushButton` 数组,你需要将数组的大小存储在一个变量中,并在需要时手动更新。
阅读全文
相关推荐
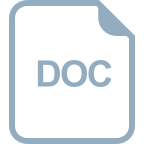
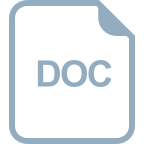
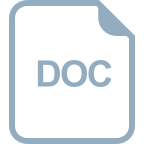
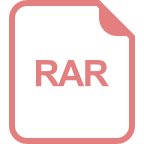
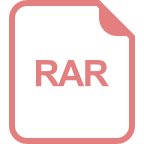











