# 创建连接和游标 conn = sqlite3.connect('logistics_center.db') cur = conn.cursor() # 创建路径表 cur.execute('''CREATE TABLE IF NOT EXISTS paths ( id INTEGER PRIMARY KEY AUTOINCREMENT, start_location TEXT NOT NULL, end_location TEXT NOT NULL, distance FLOAT NOT NULL)''') # 插入路径数据 cur.execute("INSERT INTO paths (start_location, end_location, distance) VALUES (?, ?, ?)", ('building 1, aisle 2', 'building 1, aisle 3', 100)) cur.execute("INSERT INTO paths (start_location, end_location, distance) VALUES (?, ?, ?)", ('building 1, aisle 3', 'building 2, aisle 1', 200)) # 提交事务并关闭连接 conn.commit() conn.close()
时间: 2023-12-07 14:02:28 浏览: 62
这段代码是使用 Python 中的 sqlite3 模块创建了一个名为 logistics_center.db 的数据库,并在其中创建了一个名为 paths 的表。该表包含了路径的起点、终点和距离,分别对应着 start_location、end_location 和 distance 这三个字段。接着,代码向该表中插入了两条数据,分别表示从 'building 1, aisle 2' 到 'building 1, aisle 3' 的距离为 100,以及从 'building 1, aisle 3' 到 'building 2, aisle 1' 的距离为 200。最后,代码提交了事务并关闭了连接。
相关问题
# 创建连接和游标 conn = sqlite3.connect('logistics_center.db') cur = conn.cursor() # 创建监控数据表 cur.execute('''CREATE TABLE IF NOT EXISTS monitoring_data ( id INTEGER PRIMARY KEY AUTOINCREMENT, car_no TEXT NOT NULL, location TEXT NOT NULL, time TIMESTAMP NOT NULL)''') # 插入监控数据 cur.execute("INSERT INTO monitoring_data (car_no, location, time) VALUES (?, ?, ?)", ('001', 'building 1, aisle 2', '2021-10-11 9:05:00')) cur.execute("INSERT INTO monitoring_data (car_no, location, time) VALUES (?, ?, ?)", ('001', 'building 1, aisle 3', '2021-10-11 9:20:00')) cur.execute("INSERT INTO monitoring_data (car_no, location, time) VALUES (?, ?, ?)", ('002', 'building 1, aisle 3', '2021-10-11 10:30:00')) cur.execute("INSERT INTO monitoring_data (car_no, location, time) VALUES (?, ?, ?)", ('002', 'building 2, aisle 1', '2021-10-11 10:50:00')) # 提交事务并关闭连接 conn.commit() conn.close()
这段代码是使用 Python 的 sqlite3 模块创建了一个名为 logistics_center.db 的 SQLite 数据库,并在其中创建了一个名为 monitoring_data 的数据表,用于存储物流车辆的监控数据。然后,代码插入了一些监控数据,包括车牌号、位置和时间。最后,代码提交了事务并关闭了数据库连接。这段代码的作用是将物流车辆的监控数据存储到 SQLite 数据库中,以便后续查询和分析。
import sqlite3 # 创建连接和游标 conn = sqlite3.connect('logistics_center.db') cur = conn.cursor() # 创建任务表 cur.execute('''CREATE TABLE IF NOT EXISTS tasks ( id INTEGER PRIMARY KEY AUTOINCREMENT, car_no TEXT NOT NULL, start_location TEXT NOT NULL, end_location TEXT NOT NULL, planned_start_time TIMESTAMP NOT NULL, planned_end_time TIMESTAMP NOT NULL, actual_start_time TIMESTAMP, actual_end_time TIMESTAMP, status TEXT NOT NULL DEFAULT 'planned')''') # 插入任务数据 cur.execute("INSERT INTO tasks (car_no, start_location, end_location, planned_start_time, planned_end_time) VALUES (?, ?, ?, ?, ?)", ('001', 'building 1, aisle 2', 'building 1, aisle 3', '2021-10-11 9:00:00', '2021-10-11 9:30:00')) cur.execute("INSERT INTO tasks (car_no, start_location, end_location, planned_start_time, planned_end_time) VALUES (?, ?, ?, ?, ?)", ('002', 'building 1, aisle 3', 'building 2, aisle 1', '2021-10-11 10:00:00', '2021-10-11 11:00:00')) # 提交事务并关闭连接 conn.commit() conn.close()
这段代码是在前面创建的 logistics_center.db 数据库中创建了一个名为 tasks 的任务表。该表包含了任务的编号、车辆编号、起点、终点、计划开始时间、计划结束时间、实际开始时间、实际结束时间和状态,分别对应着 id、car_no、start_location、end_location、planned_start_time、planned_end_time、actual_start_time、actual_end_time 和 status 这九个字段。其中,id 是主键,自动递增;status 的默认值为 'planned'。接着,代码向该表中插入了两条数据,分别表示编号为 '001' 的车辆从 'building 1, aisle 2' 到 'building 1, aisle 3' 的任务,计划开始时间为 '2021-10-11 9:00:00',计划结束时间为 '2021-10-11 9:30:00';编号为 '002' 的车辆从 'building 1, aisle 3' 到 'building 2, aisle 1' 的任务,计划开始时间为 '2021-10-11 10:00:00',计划结束时间为 '2021-10-11 11:00:00'。最后,代码提交了事务并关闭了连接。
阅读全文
相关推荐
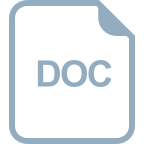
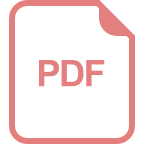




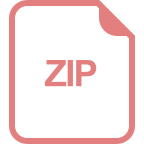
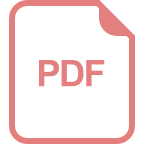
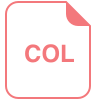






