python凯撒密码加密解密
时间: 2023-07-25 20:18:58 浏览: 32
凯撒密码是一种古老的加密方式,它采用了简单的替换方法,即将明文中的每个字母按照一定的偏移值替换成另一个字母,从而形成密文。下面是 Python 中实现凯撒密码加密和解密的示例代码:
```python
# 凯撒密码加密
def caesar_encrypt(plaintext, shift):
ciphertext = ''
for char in plaintext:
if char.isalpha():
# 将字母按照偏移值进行替换
char_code = ord(char) + shift
if char.isupper():
if char_code > ord('Z'):
char_code -= 26
elif char_code < ord('A'):
char_code += 26
else:
if char_code > ord('z'):
char_code -= 26
elif char_code < ord('a'):
char_code += 26
ciphertext += chr(char_code)
else:
ciphertext += char
return ciphertext
# 凯撒密码解密
def caesar_decrypt(ciphertext, shift):
plaintext = ''
for char in ciphertext:
if char.isalpha():
# 将字母按照偏移值进行替换
char_code = ord(char) - shift
if char.isupper():
if char_code < ord('A'):
char_code += 26
elif char_code > ord('Z'):
char_code -= 26
else:
if char_code < ord('a'):
char_code += 26
elif char_code > ord('z'):
char_code -= 26
plaintext += chr(char_code)
else:
plaintext += char
return plaintext
# 测试代码
plaintext = 'hello world'
shift = 3
ciphertext = caesar_encrypt(plaintext, shift)
print(ciphertext) # 输出: khoor zruog
decrypted_plaintext = caesar_decrypt(ciphertext, shift)
print(decrypted_plaintext) # 输出: hello world
```
在上面的示例代码中,`caesar_encrypt` 函数接受明文和偏移值作为参数,将明文中的每个字母按照偏移值进行替换,最终返回密文。`caesar_decrypt` 函数接受密文和偏移值作为参数,将密文中的每个字母按照偏移值进行替换,最终返回明文。
阅读全文
相关推荐
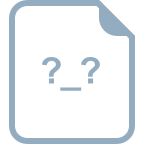
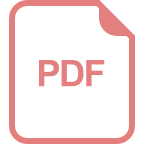
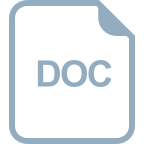
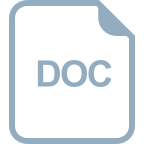






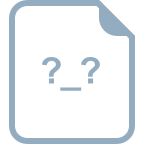