在2D平面上,使用C++,创建一条直线,并求出一点到这条直线的距离,注释语言为简体中文
时间: 2024-02-20 18:01:20 浏览: 24
以下是使用 C++ 在 2D 平面上创建一条直线,并计算一点到该直线的距离的示例代码,注释为简体中文:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 定义二维向量结构体
struct Vector2 {
double x, y;
Vector2() : x(0), y(0) {}
Vector2(double x, double y) : x(x), y(y) {}
// 向量加法
Vector2 operator+(const Vector2& other) const {
return Vector2(x + other.x, y + other.y);
}
// 向量减法
Vector2 operator-(const Vector2& other) const {
return Vector2(x - other.x, y - other.y);
}
// 向量数量积
double operator*(const Vector2& other) const {
return x * other.x + y * other.y;
}
// 向量叉积
double operator^(const Vector2& other) const {
return x * other.y - y * other.x;
}
// 向量长度
double length() const {
return sqrt(x * x + y * y);
}
};
// 定义直线结构体
struct Line {
Vector2 point1, point2;
Line() {}
Line(const Vector2& point1, const Vector2& point2) : point1(point1), point2(point2) {}
// 获取直线的一般式 Ax + By + C = 0 的系数 A、B、C
void getABC(double& A, double& B, double& C) const {
A = point2.y - point1.y;
B = point1.x - point2.x;
C = point2.x * point1.y - point1.x * point2.y;
}
// 求点到直线的距离
double distance(const Vector2& point) const {
double A, B, C;
getABC(A, B, C);
return abs(A * point.x + B * point.y + C) / sqrt(A * A + B * B);
}
};
int main() {
// 创建一条直线
Line line(Vector2(0, 0), Vector2(1, 1));
// 计算点 (2, 3) 到直线的距离
Vector2 point(2, 3);
double dist = line.distance(point);
// 输出结果
cout << "点 (" << point.x << ", " << point.y << ") 到直线的距离为:" << dist << endl;
return 0;
}
```
以上代码定义了向量和直线的结构体,并利用向量的加减法、数量积、叉积等运算,实现了计算点到直线距离的函数。在 `main` 函数中创建一条直线和一个点,并调用该函数计算出距离,最后输出结果。
相关推荐
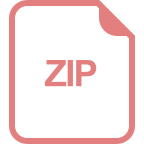
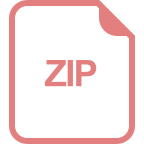
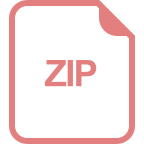














