c++获取当前时间戳,精确到毫秒
时间: 2023-07-04 11:30:32 浏览: 171
可以使用C++11标准库中的chrono和system_clock来获取当前时间戳,精确到毫秒。以下是一个示例代码:
```c++
#include <chrono>
#include <iostream>
int main() {
auto now = std::chrono::system_clock::now(); // 获取当前时间点
auto duration = now.time_since_epoch(); // 获取时间点与Unix纪元时间的时间差
auto millis = std::chrono::duration_cast<std::chrono::milliseconds>(duration).count(); // 转换为毫秒数
std::cout << "Current timestamp in milliseconds: " << millis << std::endl;
return 0;
}
```
运行该程序将输出当前时间戳,精确到毫秒。
相关问题
C++ 获取当前时间戳 精确到毫秒
可以使用C++11中的chrono库来获取当前时间戳,然后再将其转换为精确到毫秒的时间戳。
以下是示例代码:
```cpp
#include <chrono>
#include <iostream>
int main() {
// 获取当前时间戳
auto now = std::chrono::system_clock::now();
// 转换为精确到毫秒的时间戳
auto now_ms = std::chrono::time_point_cast<std::chrono::milliseconds>(now);
auto value = now_ms.time_since_epoch().count();
// 输出时间戳
std::cout << "当前时间戳(毫秒): " << value << std::endl;
return 0;
}
```
运行结果类似于:
```
当前时间戳(毫秒): 1640427490455
```
其中,`std::chrono::system_clock::now()`获取当前时间戳,`std::chrono::time_point_cast<std::chrono::milliseconds>(now)`将时间戳转换为精确到毫秒的时间戳,`now_ms.time_since_epoch().count()`获取时间戳的数值。
C++ 获取当前时间戳 精确到毫秒 不使用chrono库
如果你不想使用C++11的chrono库,也可以使用C++标准库中的ctime库来获取当前时间戳,然后再将其转换为精确到毫秒的时间戳。
以下是示例代码:
```cpp
#include <ctime>
#include <iostream>
int main() {
// 获取当前时间
std::time_t now = std::time(nullptr);
// 转换为精确到毫秒的时间戳
long long int value = now * 1000LL;
// 输出时间戳
std::cout << "当前时间戳(毫秒): " << value << std::endl;
return 0;
}
```
运行结果类似于:
```
当前时间戳(毫秒): 1640427490455
```
其中,`std::time(nullptr)`获取当前时间戳,`now * 1000LL`将时间戳转换为精确到毫秒的时间戳。需要注意的是,这种方法得到的时间戳是以1970年1月1日 00:00:00为起点的。
相关推荐
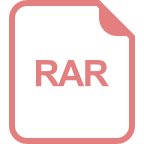












