假如我已经知道并提供了一个文件里所有字符的出现数量且按升序排列,请写一个cpp代码,实现求得其经过huffman编码后的二进制文件长度
时间: 2024-12-12 07:32:42 浏览: 3
Huffman编码是一种用于数据压缩的无损编码方式,尤其适合频率较高的字符。在C++中,我们可以利用优先队列(可以用`std::priority_queue`实现)构建Huffman树,然后生成对应的编码表。这里是一个简单的示例,假设我们已经有了一个存储字符及其频率的map:
```cpp
#include <iostream>
#include <queue>
#include <unordered_map>
#include <vector>
struct Node {
char ch;
int freq;
std::pair<Node*, Node*> children;
bool operator<(const Node& other) const { return freq < other.freq; }
};
Node* buildHuffmanTree(const std::unordered_map<char, int>& frequency) {
std::priority_queue<Node, std::vector<Node>, Node> pq(frequency.begin(), frequency.end());
while (pq.size() > 1) {
Node* left = pq.top(); pq.pop();
Node* right = pq.top(); pq.pop();
Node* newNode = new Node();
newNode->children = {left, right};
newNode->freq = left->freq + right->freq;
pq.push(newNode);
}
return pq.top();
}
std::string generateCodes(Node* root, std::string code = "") {
if (!root->children.empty()) {
return generateCodes(root->children[0], code + "0") + generateCodes(root->children[1], code + "1");
} else {
return code + root->ch;
}
}
int getBinaryLengthAfterHuffmanEncoding(const std::unordered_map<char, int>& frequency, const std::string& codes) {
std::string encodedData;
for (const auto& pair : frequency) {
encodedData += codes[pair.first];
}
// 计算总长度,每个字符由前缀码组成
int length = 0;
for (char c : encodedData) {
length += c == '1';
}
return length;
}
int main() {
// 假设有一个名为frequencies的map存储了字符及其频率
std::unordered_map<char, int> frequencies = ...; // 提供实际频率
Node* huffmanRoot = buildHuffmanTree(frequencies);
std::string codes = generateCodes(huffmanRoot);
int binaryLength = getBinaryLengthAfterHuffmanEncoding(frequencies, codes);
std::cout << "The binary file length after Huffman encoding is: " << binaryLength << std::endl;
// 清理内存
delete huffmanRoot;
return 0;
}
```
这个代码首先构建Huffman树,然后生成每个字符的编码,最后计算编码后的二进制文件长度。注意,在实际应用中,你需要提供一个存储字符频率的`frequency` map,并在main函数中填充它。
阅读全文
相关推荐
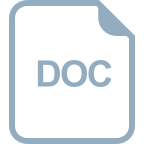
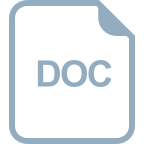
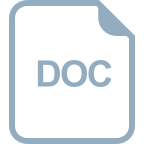
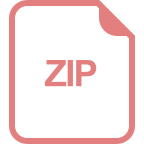
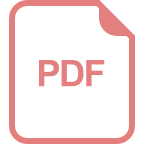
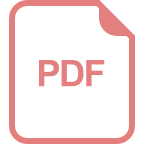
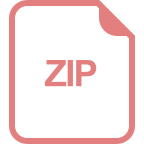
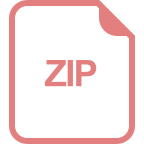
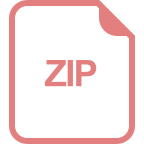
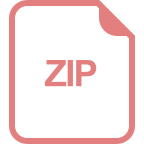
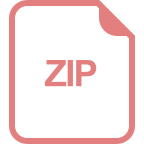
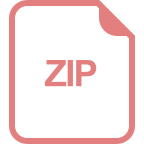
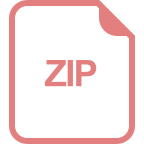
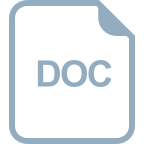
