android 如何调用OPENAI API 实现chatgpt对话,写出详细代码
时间: 2024-05-08 20:17:29 浏览: 12
作为AI语言处理技术的代表,OpenAI的GPT模型在对话系统中有着广泛的应用。在Android平台上实现ChatGPT对话,需要调用OpenAI API并进行相应的数据处理和界面交互。下面是一个简单的示例代码,供参考:
1. 添加依赖库
在build.gradle文件中添加以下依赖库:
```
dependencies {
implementation 'com.squareup.okhttp3:okhttp:4.9.0'
implementation 'com.google.code.gson:gson:2.8.6'
}
```
2. 创建ChatGPT类
在Android应用中创建ChatGPT类,实现与OpenAI API的交互,包括发送请求、解析响应等操作。以下是一个示例代码:
```
public class ChatGPT {
private String apiKey;
private String apiUrl;
private OkHttpClient client;
public ChatGPT(String apiKey, String apiUrl) {
this.apiKey = apiKey;
this.apiUrl = apiUrl;
this.client = new OkHttpClient();
}
public String getResponse(String prompt) throws IOException {
String json = "{\"prompt\":\"" + prompt + "\",\"temperature\":0.7,\"max_tokens\":60,\"top_p\":1,\"frequency_penalty\":0,\"presence_penalty\":0}";
RequestBody body = RequestBody.create(json, MediaType.parse("application/json"));
Request request = new Request.Builder()
.url(apiUrl)
.addHeader("Authorization", "Bearer " + apiKey)
.addHeader("Content-Type", "application/json")
.post(body)
.build();
Response response = client.newCall(request).execute();
String responseJson = response.body().string();
JsonObject jsonObject = JsonParser.parseString(responseJson).getAsJsonObject();
JsonArray jsonArray = jsonObject.getAsJsonArray("choices");
JsonObject choice = jsonArray.get(0).getAsJsonObject();
String text = choice.getAsJsonPrimitive("text").getAsString();
return text;
}
}
```
其中,apiKey和apiUrl分别是OpenAI API的密钥和URL。
3. 创建MainActivity类
在Android应用中创建MainActivity类,实现界面交互和对话逻辑。以下是一个示例代码:
```
public class MainActivity extends AppCompatActivity {
private EditText editText;
private TextView textView;
private ChatGPT chatGPT;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
textView = findViewById(R.id.textView);
chatGPT = new ChatGPT("your_api_key", "https://api.openai.com/v1/engines/davinci-codex/completions");
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String prompt = editText.getText().toString();
if (prompt.isEmpty()) {
return;
}
try {
String response = chatGPT.getResponse(prompt);
textView.append("You: " + prompt + "\n");
textView.append("AI: " + response + "\n\n");
editText.setText("");
} catch (IOException e) {
e.printStackTrace();
}
}
});
}
}
```
其中,editText和textView分别是输入和输出的文本框,button是发送按钮。在点击按钮时,从输入框获取对话内容,调用ChatGPT类的getResponse方法获取AI的回复,并在输出框中显示对话记录。
以上是一个简单的实现ChatGPT对话的示例代码,具体实现还需要根据具体需求进行调整和优化。
相关推荐
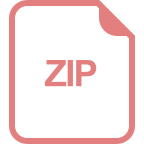
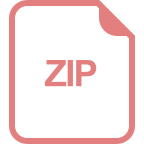
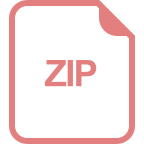














