如何使用android调用api,并写出代码
时间: 2024-05-03 20:21:58 浏览: 83
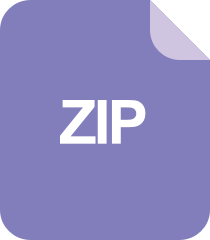
android手写识别SDK
使用Android调用API需要以下步骤:
1. 在AndroidManifest.xml文件中添加网络权限:
```
<uses-permission android:name="android.permission.INTERNET" />
```
2. 在Activity或Fragment中创建一个异步任务(AsyncTask)来执行API调用。在这个异步任务中,可以使用HttpURLConnection或OkHttp等库来发送HTTP请求并获取API响应。
3. 在异步任务的doInBackground()方法中执行API调用,例如:
```
URL url = new URL("https://api.example.com/data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
InputStream in = new BufferedInputStream(conn.getInputStream());
String response = convertStreamToString(in);
```
其中,convertStreamToString()是将输入流转换为字符串的自定义方法,例如:
```
private String convertStreamToString(InputStream is) {
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder sb = new StringBuilder();
String line;
try {
while ((line = reader.readLine()) != null) {
sb.append(line).append('\n');
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return sb.toString();
}
```
4. 在异步任务的onPostExecute()方法中处理API响应,例如:
```
protected void onPostExecute(String response) {
try {
JSONObject json = new JSONObject(response);
JSONArray data = json.getJSONArray("data");
for (int i = 0; i < data.length(); i++) {
JSONObject item = data.getJSONObject(i);
// TODO: 处理API响应数据
}
} catch (JSONException e) {
e.printStackTrace();
}
}
```
完整的示例代码如下:
```
public class MyActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
new ApiTask().execute();
}
private class ApiTask extends AsyncTask<Void, Void, String> {
@Override
protected String doInBackground(Void... voids) {
try {
URL url = new URL("https://api.example.com/data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
InputStream in = new BufferedInputStream(conn.getInputStream());
String response = convertStreamToString(in);
return response;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
@Override
protected void onPostExecute(String response) {
try {
JSONObject json = new JSONObject(response);
JSONArray data = json.getJSONArray("data");
for (int i = 0; i < data.length(); i++) {
JSONObject item = data.getJSONObject(i);
// TODO: 处理API响应数据
}
} catch (JSONException e) {
e.printStackTrace();
}
}
private String convertStreamToString(InputStream is) {
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder sb = new StringBuilder();
String line;
try {
while ((line = reader.readLine()) != null) {
sb.append(line).append('\n');
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return sb.toString();
}
}
}
```
阅读全文
相关推荐
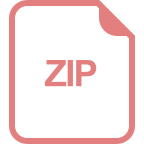
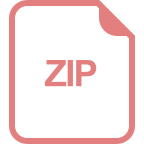


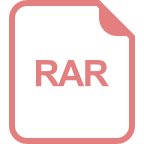
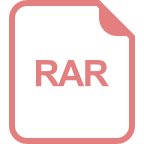
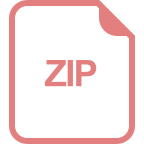
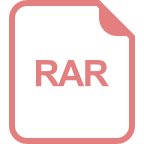
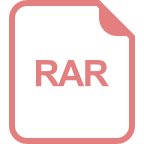
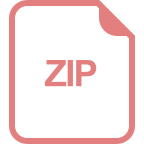
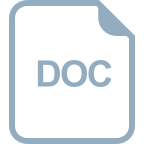





