python俄罗斯方块小游戏
时间: 2023-06-10 10:07:57 浏览: 105
好的,我来为您介绍如何使用 Python 实现俄罗斯方块小游戏。
首先,我们需要安装 Pygame,它是一个 Python 编写的游戏库,可以用来创建游戏。
1. 安装 Pygame
可以在终端输入以下命令来安装 Pygame:
```
pip install pygame
```
2. 编写游戏代码
下面是一个简单的俄罗斯方块游戏的代码示例:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 游戏窗口大小
size = (500, 600)
screen = pygame.display.set_mode(size)
# 游戏标题
pygame.display.set_caption("俄罗斯方块")
# 颜色定义
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
gray = (128, 128, 128)
# 方块大小
block_size = 20
# 游戏区域大小
play_width = 10 * block_size
play_height = 20 * block_size
# 游戏区域位置
play_x = (size[0] - play_width) // 2
play_y = size[1] - play_height - 10
# 方块形状定义
tetrominoes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6, 6, 6]],
[[7, 7],
[7, 7]]
]
# 随机生成方块
def new_piece():
shape = random.choice(tetrominoes)
piece = {}
piece["shape"] = shape
piece["color"] = random.choice([red, green, blue, gray])
piece["x"] = play_x + play_width // 2 - len(shape[0]) // 2 * block_size
piece["y"] = play_y - len(shape) * block_size
return piece
# 绘制方块
def draw_piece(piece):
shape = piece["shape"]
color = piece["color"]
x = piece["x"]
y = piece["y"]
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
pygame.draw.rect(screen, color, [x + j * block_size, y + i * block_size, block_size, block_size])
# 检测方块是否碰到边界或其他方块
def is_collision(piece, board):
shape = piece["shape"]
x = piece["x"]
y = piece["y"]
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if x + j * block_size < play_x or \
x + j * block_size >= play_x + play_width or \
y + i * block_size >= play_y + play_height or \
board[(y + i * block_size - play_y) // block_size][(x + j * block_size - play_x) // block_size] != black:
return True
return False
# 添加方块到游戏区域
def add_piece(piece, board):
shape = piece["shape"]
x = piece["x"]
y = piece["y"]
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
board[(y + i * block_size - play_y) // block_size][(x + j * block_size - play_x) // block_size] = piece["color"]
# 检测是否有完整的一行
def check_row(board):
count = 0
for i in range(len(board)):
if black not in board[i]:
count += 1
board.pop(i)
board.insert(0, [black for _ in range(10)])
return count
# 绘制游戏区域
def draw_board(board):
for i in range(len(board)):
for j in range(len(board[i])):
pygame.draw.rect(screen, board[i][j], [play_x + j * block_size, play_y + i * block_size, block_size, block_size], 0)
pygame.draw.rect(screen, white, [play_x + j * block_size, play_y + i * block_size, block_size, block_size], 1)
# 游戏主循环
def game_loop():
board = [[black for _ in range(10)] for _ in range(20)]
piece = new_piece()
clock = pygame.time.Clock()
score = 0
falling_speed = 0.5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
piece["x"] -= block_size
if is_collision(piece, board):
piece["x"] += block_size
elif event.key == pygame.K_RIGHT:
piece["x"] += block_size
if is_collision(piece, board):
piece["x"] -= block_size
elif event.key == pygame.K_DOWN:
piece["y"] += block_size
if is_collision(piece, board):
piece["y"] -= block_size
elif event.key == pygame.K_SPACE:
while not is_collision(piece, board):
piece["y"] += block_size
piece["y"] -= block_size
screen.fill(white)
# 绘制游戏区域
draw_board(board)
# 绘制方块
draw_piece(piece)
# 判断方块是否碰到底部或其他方块
if is_collision(piece, board):
add_piece(piece, board)
score += check_row(board)
piece = new_piece()
# 绘制分数
font = pygame.font.SysFont(None, 25)
text = font.render("Score: " + str(score), True, black)
screen.blit(text, (10, 10))
# 更新屏幕
pygame.display.update()
# 控制方块下落速度
clock.tick(30)
falling_speed += 0.002
pygame.time.delay(10)
piece["y"] += int(falling_speed * block_size)
# 启动游戏
game_loop()
```
3. 运行游戏
将上述代码保存为 Python 文件,然后在终端中进入该文件所在目录,输入以下命令运行游戏:
```
python 文件名.py
```
游戏窗口将会弹出,您就可以开始玩这个简单的俄罗斯方块游戏了。
相关推荐
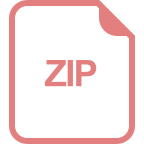










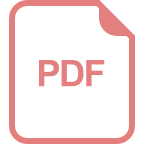