springboot写一个交易
时间: 2023-10-14 22:12:40 浏览: 33
下面是一个简单的Spring Boot交易示例,包括创建订单,更新订单和取消订单:
首先定义订单实体类Order:
```java
@Entity
@Table(name = "orders")
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String status;
@Column(nullable = false)
private Double amount;
// getter and setter methods
}
```
定义一个订单仓库接口:
```java
public interface OrderRepository extends JpaRepository<Order, Long> {
}
```
创建订单的控制器:
```java
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private OrderRepository orderRepository;
@PostMapping
public Order createOrder(@RequestBody Order order) {
order.setStatus("created");
return orderRepository.save(order);
}
@PutMapping("/{id}")
public Order updateOrder(@PathVariable Long id, @RequestBody Order order) {
Order existingOrder = orderRepository.findById(id).orElseThrow(() -> new RuntimeException("Order not found"));
existingOrder.setStatus(order.getStatus());
existingOrder.setAmount(order.getAmount());
return orderRepository.save(existingOrder);
}
@DeleteMapping("/{id}")
public void cancelOrder(@PathVariable Long id) {
Order existingOrder = orderRepository.findById(id).orElseThrow(() -> new RuntimeException("Order not found"));
existingOrder.setStatus("cancelled");
orderRepository.save(existingOrder);
}
}
```
这个控制器定义了三个HTTP端点,用于创建、更新和取消订单。在创建订单时,订单的状态设置为“created”,在取消订单时,订单的状态设置为“cancelled”。
在启动应用程序之前,需要在application.properties文件中配置数据库连接和Hibernate JPA:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=root
spring.datasource.password=mypassword
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
```
启动应用程序后,可以使用curl命令或Postman发送HTTP请求来测试这些端点。例如,要创建一个新订单,可以发送以下POST请求:
```
POST http://localhost:8080/orders
{
"status": "created",
"amount": 100.0
}
```
响应将返回包含新订单的JSON对象:
```
{
"id": 1,
"status": "created",
"amount": 100.0
}
```
要更新订单,可以发送以下PUT请求:
```
PUT http://localhost:8080/orders/1
{
"status": "paid",
"amount": 150.0
}
```
响应将返回更新后的订单:
```
{
"id": 1,
"status": "paid",
"amount": 150.0
}
```
要取消订单,可以发送以下DELETE请求:
```
DELETE http://localhost:8080/orders/1
```
响应将不包含任何内容。
相关推荐
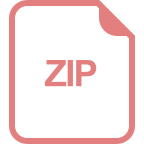
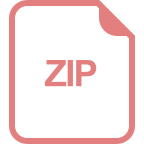
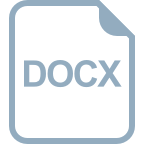













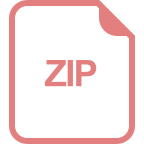