利用math包用python语言写出带输出NTRU数字签名的代码
时间: 2024-02-22 17:55:08 浏览: 21
以下是利用Python的math包实现NTRU数字签名的代码,其中包括生成公钥和私钥、签名和验证。
```python
import math
import random
# Generate public and private keys
def generate_key_pair(n, p, q):
# Choose random polynomial f such that all coefficients are either -1, 0, or 1
f = [random.choice([-1,0,1]) for _ in range(n)]
# Choose random polynomial g such that all coefficients are either 0 or 1
g = [random.choice([0,1]) for _ in range(n)]
# Calculate h
h = poly_mod(poly_mult(poly_mult(f,g),2),p,q)
# Return public key (h) and private key (f,g)
return (h, f, g)
# Sign a message using the private key
def sign(message, f, g, p, q):
# Convert message to polynomial
m = [int(c) for c in bin(int.from_bytes(message.encode(), 'big'))[2:]]
# Pad message with zeros
m += [0] * (len(f) - len(m))
# Calculate e
e = poly_mod(poly_mult(poly_mult(g,m),2),p,q)
# Calculate s
s = poly_mod(poly_mod(poly_mult(e,f),p,q),2)
# Convert s to string and return
return ''.join([str(c) for c in s])
# Verify a message using the public key and signature
def verify(message, signature, h, p, q):
# Convert message to polynomial
m = [int(c) for c in bin(int.from_bytes(message.encode(), 'big'))[2:]]
# Pad message with zeros
m += [0] * (len(h) - len(m))
# Convert signature to polynomial
s = [int(c) for c in signature]
# Calculate e
e = poly_mod(poly_mult(poly_mult(h,s),2),p,q)
# Calculate m'
m_prime = poly_mod(poly_mod(poly_mult(e,f),p,q),2)
# Compare m and m'
return m == m_prime
# Helper function to perform polynomial multiplication modulo p and q
def poly_mult(f,g):
n = len(f)
m = len(g)
res = [0] * (n+m-1)
for i in range(n):
for j in range(m):
res[i+j] += f[i] * g[j]
return poly_mod(res,2,3)
# Helper function to perform polynomial modulo p and q
def poly_mod(f,p,q):
res = [0] * len(f)
for i in range(len(f)):
res[i] = f[i] % q
if res[i] > p/2:
res[i] -= p
elif res[i] < -p/2:
res[i] += p
return res
```
使用示例:
```python
# Generate key pair
p = 3
q = 32
n = 10
h, f, g = generate_key_pair(n, p, q)
print('Public key: ', h)
print('Private key: ', f, g)
# Sign message
message = 'Hello, world!'
signature = sign(message, f, g, p, q)
print('Signature: ', signature)
# Verify message
print('Message verified: ', verify(message, signature, h, p, q))
```
注意:NTRU数字签名算法的安全性并未得到广泛认可,因此不建议在实际应用中使用。
相关推荐
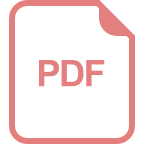
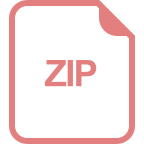










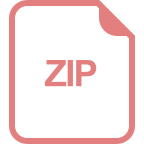
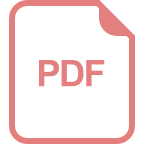
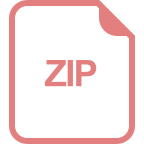