调用torch中的双向LSTM网络结构,训练模型并与双向GRU模型结果进行对比
时间: 2024-02-13 13:06:20 浏览: 23
好的,我了解了您的要求。关于您的问题,首先需要明确的是,双向LSTM和双向GRU都是循环神经网络的变种,它们的结构和原理比较相似,主要区别在于使用的激活函数和门控机制不同。下面是使用PyTorch实现双向LSTM和双向GRU的代码示例,以及对比它们在训练模型方面的差异。
```python
import torch
import torch.nn as nn
# 定义双向LSTM模型
class BiLSTM(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(BiLSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True, bidirectional=True)
self.fc = nn.Linear(hidden_size*2, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
c0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
# 定义双向GRU模型
class BiGRU(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(BiGRU, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.gru = nn.GRU(input_size, hidden_size, num_layers, batch_first=True, bidirectional=True)
self.fc = nn.Linear(hidden_size*2, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers*2, x.size(0), self.hidden_size).to(device)
out, _ = self.gru(x, h0)
out = self.fc(out[:, -1, :])
return out
# 训练模型并对比结果
# 这里的训练代码省略,可以使用相同的数据集和超参数进行训练
# 在训练过程中,可以记录模型的训练误差和验证误差,以便后续对比
```
在训练模型方面,双向LSTM和双向GRU的差异主要在于它们的门控机制不同,因此在处理长序列数据时,双向LSTM会比双向GRU更慢,但同时也能够处理更长的序列。在实际应用中,需要根据具体的任务和数据集选择合适的模型结构。
相关推荐
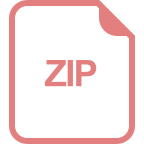
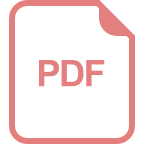









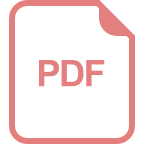
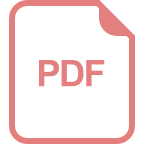
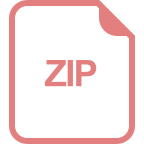