帮我写个C++视频转码服务
时间: 2023-03-04 11:56:04 浏览: 67
视频转码服务是一个常见的网络应用程序,它可以将不同格式的视频文件转换为需要的格式。在本文中,我将提供一个简单的C语言实现,该实现使用FFmpeg库来进行视频转码。
首先,你需要安装FFmpeg库。你可以在 https://ffmpeg.org/ 下载FFmpeg。安装完成后,你需要包含FFmpeg的头文件,并链接FFmpeg的库文件。
接下来,我们可以开始编写代码。下面是一个简单的C程序,它可以将输入文件转换为输出文件。
```
#include <stdio.h>
#include <stdlib.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
int main(int argc, char **argv)
{
if (argc < 3) {
fprintf(stderr, "Usage: %s <input_file> <output_file>\n", argv[0]);
return 1;
}
const char *input_file = argv[1];
const char *output_file = argv[2];
av_register_all();
AVFormatContext *format_ctx = NULL;
if (avformat_open_input(&format_ctx, input_file, NULL, NULL) < 0) {
fprintf(stderr, "Could not open input file '%s'\n", input_file);
return 1;
}
if (avformat_find_stream_info(format_ctx, NULL) < 0) {
fprintf(stderr, "Could not find stream information\n");
return 1;
}
av_dump_format(format_ctx, 0, input_file, 0);
int video_stream_index = -1;
for (int i = 0; i < format_ctx->nb_streams; i++) {
if (format_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
fprintf(stderr, "Could not find video stream\n");
return 1;
}
AVCodecParameters *codec_params = format_ctx->streams[video_stream_index]->codecpar;
AVCodec *codec = avcodec_find_decoder(codec_params->codec_id);
if (!codec) {
fprintf(stderr, "Codec not found\n");
return 1;
}
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Could not allocate codec context\n");
return 1;
}
if (avcodec_parameters_to_context(codec_ctx, codec_params) < 0) {
fprintf(stderr, "Could not initialize codec context\n");
return 1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
return 1;
}
AVFrame *frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Could not allocate frame\n");
return 1;
}
AVPacket packet;
av_init_packet(&packet);
FILE *output_file_handle = fopen(output_file, "wb");
if (!output_file_handle) {
fprintf(stderr, "Could not open output file '%s'\n", output_file);
return 1;
}
int frame_count = 0;
while (av_read_frame(format_ctx, &packet) >= 0) {
if (packet.stream_index == video_stream
相关推荐
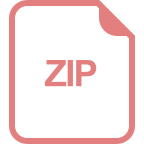
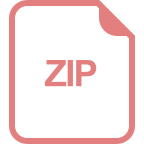














