)PrintList():输出显示有序单循环链表La和Lb。比如H->1>3->6->8->10:的c语言代码
时间: 2024-09-22 12:02:53 浏览: 84
在C语言中,编写一个名为`PrintList()`的函数来输出显示两个有序单循环链表(La和Lb),你可以按照节点数据的顺序依次打印出来。下面是一个简单的示例,假设这两个链表都是整数类型的,并且它们都有相同的结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 在链表末尾添加节点
void append(Node** head, int value) {
Node* newNode = createNode(value);
if (*head == NULL) {
*head = newNode;
} else {
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 输出链表中的所有元素
void PrintList(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
// 主函数示例,假设La 和 Lb 已经初始化
int main() {
// 初始化两个有序链表
Node* La = NULL;
append(&La, 1);
append(&La, 3);
append(&La, 6);
append(&La, 8);
append(&La, 10);
Node* Lb = NULL;
append(&Lb, 2);
append(&Lb, 4);
append(&Lb, 5);
append(&Lb, 7);
append(&Lb, 9);
printf("List La: ");
PrintList(La);
printf("List Lb: ");
PrintList(Lb);
return 0;
}
```
这段代码首先定义了链表节点结构体,并提供了一个`PrintList()`函数用于打印链表内容。在`main()`函数中,我们分别创建了两个有序链表La和Lb,并通过`append()`函数添加节点。然后分别调用`PrintList()`函数打印它们。
当运行此程序,它会输出类似这样的结果:
```
List La: 1 -> 3 -> 6 -> 8 -> 10 ->
List Lb: 2 -> 4 -> 5 -> 7 -> 9 ->
```
阅读全文
相关推荐
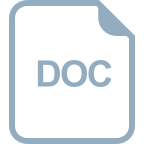
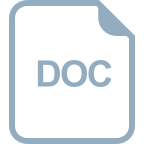
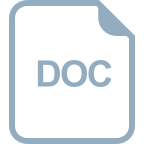
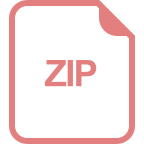
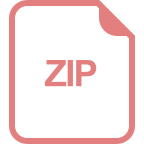
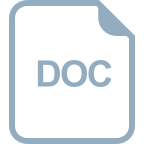
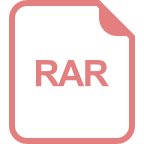
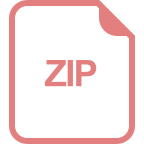
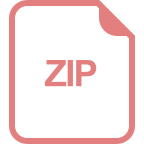
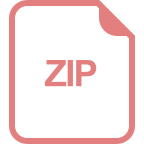
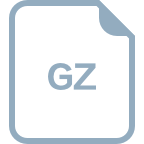
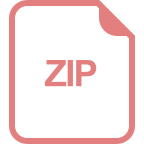
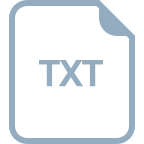
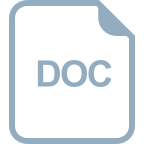
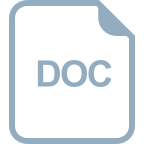
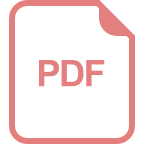
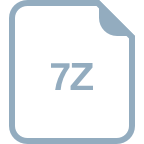