python需求预测
时间: 2023-11-22 10:49:58 浏览: 47
根据提供的引用内容,可以使用Python进行需求预测。以下是两种常见的预测方法:
1. 递归多步预测:该方法需要进行递归预测,其中每个新预测都基于前一个预测。可以使用ARIMA模型进行预测,该模型可以自动处理时间序列数据中的趋势和季节性。具体步骤如下:
```python
from statsmodels.tsa.arima_model import ARIMA
import pandas as pd
# 读取数据
data = pd.read_csv('power_demand.csv', header=0, index_col=0, parse_dates=True, squeeze=True)
# 拟合ARIMA模型
model = ARIMA(data, order=(5,1,0))
model_fit = model.fit(disp=0)
# 递归预测未来n个时间步长
n = 24 # 预测未来24小时的需求
forecast = model_fit.forecast(steps=n)[0]
print(forecast)
```
2. 直接多步预测:该方法涉及为每个步骤训练不同的模型。可以使用神经网络模型进行预测,该模型可以自动学习时间序列数据中的复杂模式。具体步骤如下:
```python
from keras.models import Sequential
from keras.layers import Dense, LSTM
import pandas as pd
import numpy as np
# 读取数据
data = pd.read_csv('power_demand.csv', header=0, index_col=0, parse_dates=True, squeeze=True)
# 将数据转换为监督学习问题
def series_to_supervised(data, n_in=1, n_out=1, dropnan=True):
n_vars = 1 if type(data) is list else data.shape[1]
df = pd.DataFrame(data)
cols, names = list(), list()
# 输入序列 (t-n, ... t-1)
for i in range(n_in, 0, -1):
cols.append(df.shift(i))
names += [('var%d(t-%d)' % (j+1, i)) for j in range(n_vars)]
# 输出序列 (t, t+1, ... t+n-1)
for i in range(0, n_out):
cols.append(df.shift(-i))
if i == 0:
names += [('var%d(t)' % (j+1)) for j in range(n_vars)]
else:
names += [('var%d(t+%d)' % (j+1, i)) for j in range(n_vars)]
# 将列拼接在一起
agg = pd.concat(cols, axis=1)
agg.columns = names
# 删除NaN行
if dropnan:
agg.dropna(inplace=True)
return agg
# 将数据转换为监督学习问题
data = series_to_supervised(data, n_in=24, n_out=24)
# 分割数据集为训练集和测试集
values = data.values
n_train_hours = 365*24*2 # 使用前两年的数据作为训练集
train = values[:n_train_hours, :]
test = values[n_train_hours:, :]
# 将数据集转换为输入和输出
train_X, train_y = train[:, :-24], train[:, -24:]
test_X, test_y = test[:, :-24], test[:, -24:]
# 将输入数据重塑为3D格式 [样本数, 时间步长, 特征数]
train_X = train_X.reshape((train_X.shape[0], 24, 1))
test_X = test_X.reshape((test_X.shape[0], 24, 1))
# 定义和拟合LSTM模型
model = Sequential()
model.add(LSTM(50, input_shape=(24, 1)))
model.add(Dense(24))
model.compile(loss='mae', optimizer='adam')
model.fit(train_X, train_y, epochs=50, batch_size=72, verbose=0)
# 预测未来n个时间步长
n = 24 # 预测未来24小时的需求
forecast = []
for i in range(n):
x_input = np.array(test_X[-1, :, :]).reshape((1, 24, 1))
yhat = model.predict(x_input, verbose=0)
forecast.append(yhat[0])
test_X = np.concatenate((test_X, yhat.reshape((1, 24, 1))), axis=1)
print(forecast)
```
相关推荐
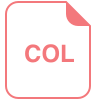
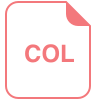
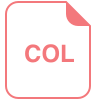
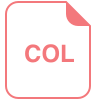
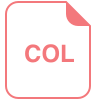









