stm32f103r6串口发送和接收指令串口助手输入a点亮b熄灭代码
时间: 2024-03-25 09:35:43 浏览: 13
下面是一个简单的示例代码,使用USART1串口模块发送和接收指令,当接收到字符'a'时点亮LED,当接收到字符'b'时熄灭LED。
```c
#include "stm32f10x.h"
void USART1_Init(void);
void LED_Init(void);
void USART1_SendChar(char ch);
char USART1_ReceiveChar(void);
int main(void)
{
char cmd;
USART1_Init();
LED_Init();
while(1)
{
cmd = USART1_ReceiveChar();
if(cmd == 'a')
{
GPIO_SetBits(GPIOA, GPIO_Pin_0); // 点亮LED
}
else if(cmd == 'b')
{
GPIO_ResetBits(GPIOA, GPIO_Pin_0); // 熄灭LED
}
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// USART1 Tx (PA9) pin configuration
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1 Rx (PA10) pin configuration
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// USART1 configuration
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
}
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// Configure LED (PA0) pin
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Turn off LED
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
}
void USART1_SendChar(char ch)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, ch);
}
char USART1_ReceiveChar(void)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
```
在使用串口助手发送字符时,需要选择9600波特率,8位数据位,无奇偶校验位,1位停止位。
相关推荐
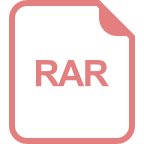
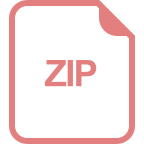
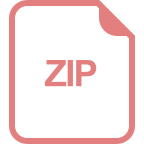














