use C program Check whether two words are Anagrams. If they are, return 1, otherwise return 0. If two words contain the same letters in different order, they are called anagram. For example, "silent" and "listen" are anagram. Input There are two lines of input, corresponding to two words. Output If two words are anagrams, output true; otherwise, output false.
时间: 2024-02-29 22:51:09 浏览: 61
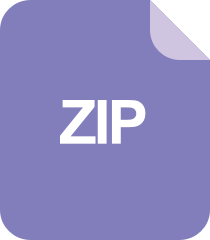
Webolith:Aerolith 2.0-用于网络的Aerolith。 单词学习网站-学习拼字游戏,Boggle,用Frentz单词学习等
Certainly! Here's a C program to check whether two words are anagrams:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[100], str2[100];
int len1, len2, i, j, freq1[26] = {0}, freq2[26] = {0};
printf("Enter first word: ");
scanf("%s", str1);
printf("Enter second word: ");
scanf("%s", str2);
len1 = strlen(str1);
len2 = strlen(str2);
if(len1 != len2) {
printf("false\n");
return 0;
}
for(i=0; i<len1; i++) {
freq1[str1[i]-'a']++;
freq2[str2[i]-'a']++;
}
for(i=0; i<26; i++) {
if(freq1[i] != freq2[i]) {
printf("false\n");
return 0;
}
}
printf("true\n");
return 0;
}
```
The program first declares two character arrays `str1` and `str2` of size 100 to store the user input. It prompts the user to enter the two words using the `printf()` and `scanf()` functions.
The program then computes the length of each word using the `strlen()` function and compares the lengths. If the lengths are not equal, the program prints "false" and exits.
If the lengths are equal, the program initializes two arrays `freq1` and `freq2` of size 26 to keep track of the frequency of each letter in each word. It then loops through each character in both words and increments the corresponding frequency array.
Finally, the program loops through the frequency arrays and checks if the frequencies of each letter in both words are equal. If they are not equal, the program prints "false". Otherwise, it prints "true".
I hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
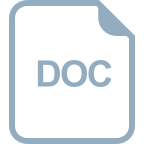
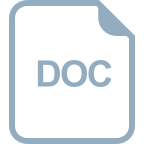
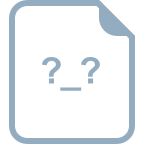
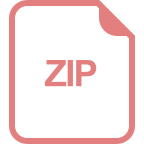
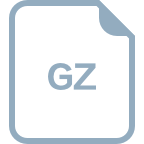
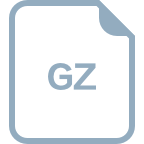
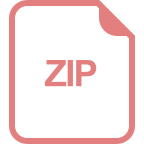
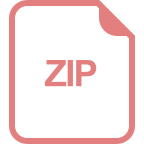
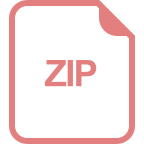
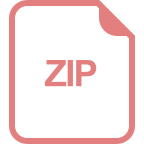




