leetcode:Group Anagrams 用c语言,只给代码
时间: 2024-05-19 16:14:09 浏览: 23
这是一个用C语言实现的LeetCode题目:Group Anagrams。
题目描述:
给定一个字符串数组,将其分组,使得包含相同字符的字符串在同一组中。返回结果应按照字母顺序排列。
示例:
输入: ["eat", "tea", "tan", "ate", "nat", "bat"]
输出:
[
["ate","eat","tea"],
["nat","tan"],
["bat"]
]
解题思路:
- 遍历字符串数组,将每个字符串转换为字符数组并排序。
- 使用哈希表将排序后相同的字符串分组。
- 遍历哈希表,将每个分组的字符串数组添加到结果数组中。
代码实现:
/**
* Note: The returned array must be malloced, assume caller calls free().
*/
char *** groupAnagrams(char ** strs, int strsSize, int* returnSize, int** returnColumnSizes){
char ***res = (char ***)malloc(sizeof(char **) * strsSize);
*returnSize = 0;
*returnColumnSizes = (int *)malloc(sizeof(int) * strsSize);
int cnt[26] = {0};
int index = 0;
struct Node *hashTable[strsSize];
memset(hashTable, 0, sizeof(hashTable));
for (int i = 0; i < strsSize; i++) {
memset(cnt, 0, sizeof(cnt));
for (int j = 0; j < strlen(strs[i]); j++) {
cnt[strs[i][j] - 'a']++;
}
char *key = (char *)malloc(sizeof(char) * 27);
int idx = 0;
for (int j = 0; j < 26; j++) {
if (cnt[j] != 0) {
key[idx++] = j + 'a';
key[idx++] = cnt[j] + '0';
}
}
key[idx] = '\0';
int hash = getHash(key);
if (hashTable[hash] == NULL) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->key = key;
node->next = NULL;
node->strings = (char **)malloc(sizeof(char *));
node->strings[0] = strs[i];
node->count = 1;
hashTable[hash] = node;
} else {
struct Node *p = hashTable[hash];
while (p != NULL) {
if (strcmp(p->key, key) == 0) {
p->count++;
p->strings = (char **)realloc(p->strings, sizeof(char *) * p->count);
p->strings[p->count - 1] = strs[i];
break;
}
p = p->next;
}
if (p == NULL) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->key = key;
node->next = hashTable[hash];
node->strings = (char **)malloc(sizeof(char *));
node->strings[0] = strs[i];
node->count = 1;
hashTable[hash] = node;
}
}
}
for (int i = 0; i < strsSize; i++) {
if (hashTable[i] != NULL) {
struct Node *p = hashTable[i];
while (p != NULL) {
res[index] = p->strings;
(*returnColumnSizes)[index] = p->count;
(*returnSize)++;
p = p->next;
index++;
}
}
}
return res;
}
int getHash(char *key) {
int hash = 0;
for (int i = 0; i < strlen(key); i++) {
hash = (hash * 31 + key[i]) % 100000;
}
return hash;
}
struct Node {
char *key;
struct Node *next;
char **strings;
int count;
};
相关推荐
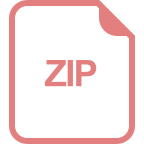
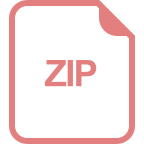














