编写一个C程序来实现shell框架
时间: 2024-11-13 12:39:29 浏览: 4
编写一个简单的shell框架涉及到创建命令解析、处理输入、以及基本的I/O操作。下面是一个非常基础的C程序示例,它使用了经典的readline库来获取用户输入,并尝试执行一些基本的命令。请注意,这只是一个简化的框架,实际的shell需要更复杂的功能和错误处理。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include "readline/readline.h"
#include "history/history.h"
// 定义一个简单的命令列表
struct command {
char* name;
void (*func)(char* args);
};
void print_help() {
printf("可用命令:\n");
// 这里可以添加更多的命令
printf("\tls\n\tcd\n");
}
void do_ls(char* args) {
system("ls");
}
void do_cd(char* args) {
if (chdir(args) != 0) {
perror("cd failed");
}
}
int main() {
struct command commands[] = {
{"ls", do_ls},
{"cd", do_cd},
{NULL, NULL} // 结束标记
};
while (true) {
char* line = readline("> ");
add_history(line); // 添加到历史记录
if (!strcmp(line, "exit")) break; // 检查是否退出
free(line);
for (struct command *cmd = commands; cmd->name; cmd++) {
if (strcmp(cmd->name, line) == 0) {
cmd->func(line);
break;
}
}
if (!cmd->name) {
print_help(); // 如果没有匹配的命令,打印帮助信息
}
}
return 0;
}
```
阅读全文
相关推荐
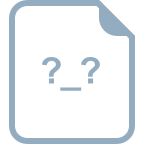
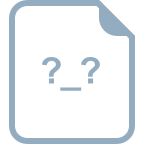
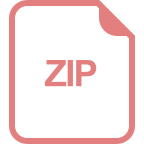

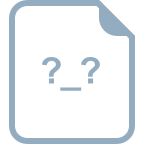
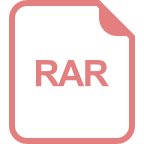
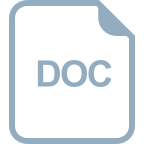

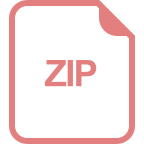
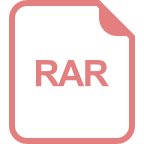
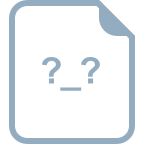
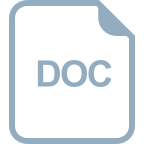
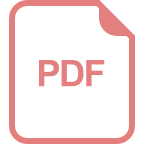
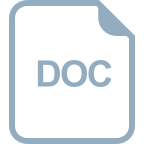
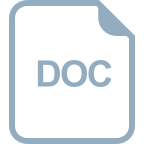
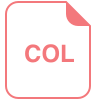
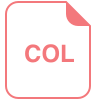


