编写一个C程序来实现shell框架并给出操作结果
时间: 2024-11-12 09:33:19 浏览: 14
编写一个完整的shell程序是一个复杂的任务,涉及到命令解析、输入处理、管道和重定向等系统调用。以下是一个简单的示例,展示了如何在C语言中创建一个基础的shell命令解释器,它只能执行一些基本的内置命令,如"echo":
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MAX_CMD_LEN 100
void execute_command(char *cmd) {
if (strcmp(cmd, "echo") == 0) {
char args[MAX_CMD_LEN];
printf("Enter command arguments: ");
fgets(args, MAX_CMD_LEN, stdin);
args[strcspn(args, "\n")] = '\0'; // remove newline at the end
printf("Echoing: %s\n", args);
} else {
printf("Unsupported command: %s\n", cmd);
}
}
int main() {
char input_cmd[MAX_CMD_LEN];
while (fgets(input_cmd, sizeof(input_cmd), stdin)) {
if (input_cmd[0] != '#') { // ignore comments starting with '#'
execute_command(input_cmd);
}
}
return 0;
}
```
这个程序首先读取用户从标准输入输入的一行命令,然后检查是否是支持的内置"echo"命令。如果不是,则返回一条错误信息。
然而,真正的shell还需要处理更复杂的情况,比如分词、处理特殊字符、使用环境变量、处理命令和参数的组合、以及执行外部命令等。此外,为了安全性和功能完整,你通常会使用更专业的工具,如POSIX shell API或libexec库。
阅读全文
相关推荐






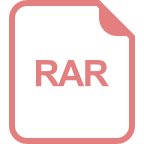



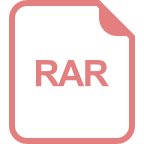







