设计并实现一个C++联系人类:contact,该类有name,tel和postcode三个私有属性,为该类设计若干方法,包括构造函数、存取三个私有属性的函数、显示联系人信息的函数等。 (2)设计并实现一个通讯录类:addressBook,该类包含一个存放所有联系人的容器。 (3)为addressBook设计若干方法,包括显示所有联系人、增加联系人、删除联系人、插入联系人、查找联系人等函数,以及将所有联系人保存到文件和从文件中读取联系人的函数。 (4)设计主函数,显示操作选择菜单,完成通讯录的显示、增加、查找、删除和保存等功能。其中,类的成员函数的定义与实现应分开在两个文件,并且禁止使用vector。应使用string类
时间: 2023-05-16 21:07:47 浏览: 135
以下是C知道的回答:
1. 以下是实现C联系人类contact的代码:
```c++
#include <string>
class contact {
private:
std::string name;
std::string tel;
std::string postcode;
public:
contact(std::string name, std::string tel, std::string postcode) {
this->name = name;
this->tel = tel;
this->postcode = postcode;
}
std::string getName() {
return name;
}
std::string getTel() {
return tel;
}
std::string getPostcode() {
return postcode;
}
void setName(std::string name) {
this->name = name;
}
void setTel(std::string tel) {
this->tel = tel;
}
void setPostcode(std::string postcode) {
this->postcode = postcode;
}
void display() {
std::cout << "Name: " << name << std::endl;
std::cout << "Tel: " << tel << std::endl;
std::cout << "Postcode: " << postcode << std::endl;
}
};
```
2. 以下是实现通讯录类addressBook的代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <list>
#include "contact.h"
class addressBook {
private:
std::list<contact> contacts;
public:
void displayAll() {
for (auto it = contacts.begin(); it != contacts.end(); it++) {
it->display();
std::cout << std::endl;
}
}
void add(contact c) {
contacts.push_back(c);
}
void remove(std::string name) {
for (auto it = contacts.begin(); it != contacts.end(); it++) {
if (it->getName() == name) {
contacts.erase(it);
break;
}
}
}
void insert(int index, contact c) {
auto it = contacts.begin();
std::advance(it, index);
contacts.insert(it, c);
}
contact find(std::string name) {
for (auto it = contacts.begin(); it != contacts.end(); it++) {
if (it->getName() == name) {
return *it;
}
}
return contact("", "", "");
}
void saveToFile(std::string filename) {
std::ofstream file(filename);
for (auto it = contacts.begin(); it != contacts.end(); it++) {
file << it->getName() << "," << it->getTel() << "," << it->getPostcode() << std::endl;
}
file.close();
}
void loadFromFile(std::string filename) {
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
std::string name, tel, postcode;
std::stringstream ss(line);
std::getline(ss, name, ',');
std::getline(ss, tel, ',');
std::getline(ss, postcode, ',');
contacts.push_back(contact(name, tel, postcode));
}
file.close();
}
};
```
3. 以下是主函数的代码:
```c++
#include <iostream>
#include <string>
#include "addressBook.h"
int main() {
addressBook book;
while (true) {
std::cout << "1. Display all contacts" << std::endl;
std::cout << "2. Add a contact" << std::endl;
std::cout << "3. Remove a contact" << std::endl;
std::cout << "4. Insert a contact" << std::endl;
std::cout << "5. Find a contact" << std::endl;
std::cout << "6. Save to file" << std::endl;
std::cout << "7. Load from file" << std::endl;
std::cout << "8. Exit" << std::endl;
int choice;
std::cin >> choice;
switch (choice) {
case 1:
book.displayAll();
break;
case 2: {
std::string name, tel, postcode;
std::cout << "Enter name: ";
std::cin >> name;
std::cout << "Enter tel: ";
std::cin >> tel;
std::cout << "Enter postcode: ";
std::cin >> postcode;
book.add(contact(name, tel, postcode));
break;
}
case 3: {
std::string name;
std::cout << "Enter name: ";
std::cin >> name;
book.remove(name);
break;
}
case 4: {
int index;
std::string name, tel, postcode;
std::cout << "Enter index: ";
std::cin >> index;
std::cout << "Enter name: ";
std::cin >> name;
std::cout << "Enter tel: ";
std::cin >> tel;
std::cout << "Enter postcode: ";
std::cin >> postcode;
book.insert(index, contact(name, tel, postcode));
break;
}
case 5: {
std::string name;
std::cout << "Enter name: ";
std::cin >> name;
contact c = book.find(name);
if (c.getName() == "") {
std::cout << "Contact not found" << std::endl;
} else {
c.display();
}
break;
}
case 6: {
std::string filename;
std::cout << "Enter filename: ";
std::cin >> filename;
book.saveToFile(filename);
break;
}
case 7: {
std::string filename;
std::cout << "Enter filename: ";
std::cin >> filename;
book.loadFromFile(filename);
break;
}
case 8:
return 0;
default:
std::cout << "Invalid choice" << std::endl;
break;
}
}
}
```
希望这些代码能够帮助你实现通讯录功能。
相关推荐
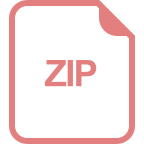
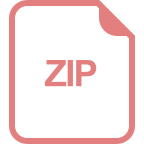
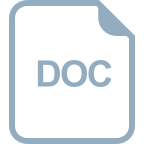
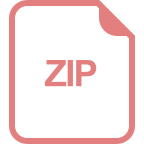
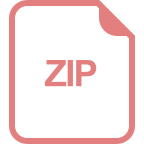
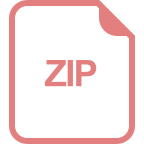
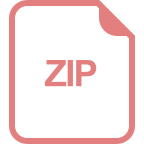
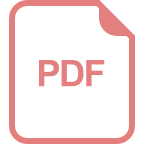
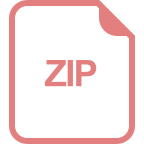
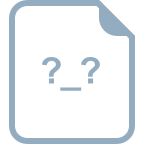
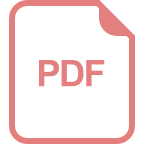
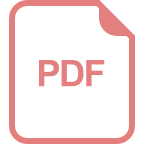
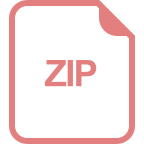
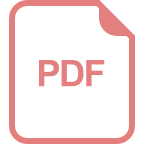
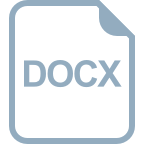
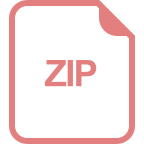