如何用Python中的循环结构计算今天是第几天
时间: 2023-09-20 11:06:10 浏览: 102
### 回答1:
以下是Python代码,可以计算今天是一年中的第几天:
```python
import datetime
today = datetime.date.today()
start_of_year = datetime.date(today.year, 1, 1)
day_of_year = (today - start_of_year).days + 1
print("今天是一年中的第", day_of_year, "天")
```
这个程序使用了Python的datetime模块来获取今天的日期,并计算出一年中的第几天。
### 回答2:
要计算今天是今年的第几天,可以使用Python中的循环结构。以下是一个简单的示例代码:
```python
import datetime
# 获取今天的日期
today = datetime.date.today()
# 初始化一个变量,用于记录天数
day_count = 0
# 使用循环结构逐月计算天数并累加
for month in range(1, today.month):
# 获取当前月份的天数
days_in_month = (datetime.date(today.year, month + 1, 1) - datetime.date(today.year, month, 1)).days
# 累加天数
day_count += days_in_month
# 加上当月的天数,即为今天是今年的第几天
day_count += today.day
print("今天是今年的第{}天。".format(day_count))
```
该代码使用datetime模块获取今天的日期,并利用循环结构逐月累加天数。最后输出结果,显示今天是今年的第几天。
### 回答3:
要使用Python中的循环结构计算今天是第几天,你可以按以下步骤进行操作:
1. 首先,导入`datetime`模块,这个模块包含了处理日期和时间的函数和类。
2. 使用`datetime.today()`函数获取当前日期。
3. 创建一个列表`month_days`,包含每个月的天数,考虑平年和闰年的情况。
4. 使用`for`循环遍历当前日期的年份之前的每一年。在循环中,对于每一年,根据是否是闰年,将一年的总天数加到计算总天数的变量中。
5. 使用`for`循环遍历当前日期的月份之前的每个月。在循环中,根据每个月的天数,将该月的天数加到计算总天数的变量中。
6. 将当前日期的天数加到计算总天数的变量中。
7. 输出计算总天数的结果。
下面是一个示例代码:
```python
import datetime
def calculate_day_of_year():
today = datetime.date.today()
year = today.year
month = today.month
day = today.day
month_days = [31,28,31,30,31,30,31,31,30,31,30,31]
total_days = 0
for y in range(year-1):
if (y % 4 == 0 and y % 100 != 0) or (y % 400 == 0):
total_days += 366
else:
total_days += 365
for m in range(month-1):
total_days += month_days[m]
total_days += day
print(f"今天是今年的第{total_days}天。")
calculate_day_of_year()
```
这段代码首先使用`datetime`模块获取当前日期。然后,使用两个`for`循环遍历年份和月份之前的日期,并根据每个月的天数计算总天数。最后,将当前日期的天数加到计算总天数的结果中,并通过`print()`函数输出结果。
阅读全文
相关推荐
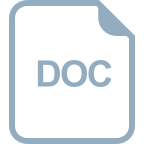
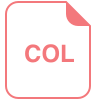
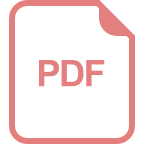
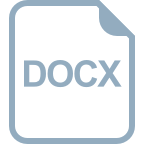
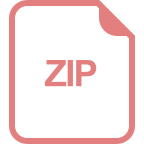
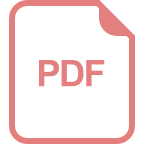
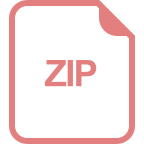
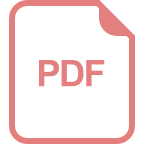
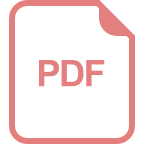
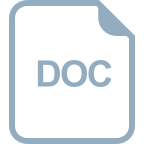
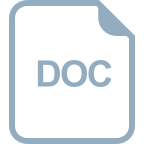
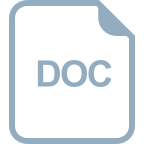
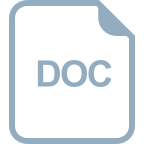
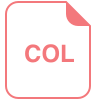
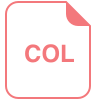
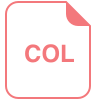
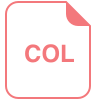