解释这段代码Consumer<LambdaQueryWrapper<SysDepart>> square = i -> { if (oConvertUtils.isNotEmpty(ids)) { if (CommonConstant.DEPART_KEY_ORG_CODE.equals(primaryKey)) { i.in(SysDepart::getOrgCode, ids.split(SymbolConstant.COMMA)); } else { i.in(SysDepart::getId, ids.split(SymbolConstant.COMMA)); } } else { if(oConvertUtils.isEmpty(parentId)){ i.and(q->q.isNull(true,SysDepart::getParentId).or().eq(true,SysDepart::getParentId,"")); }else{ i.eq(true,SysDepart::getParentId,parentId); } } };
时间: 2024-04-11 09:33:15 浏览: 8
这段代码定义了一个 Consumer 对象,它接受一个 LambdaQueryWrapper<SysDepart> 参数。Consumer 是一个函数式接口,它有一个抽象方法 accept,可以接受一个输入参数并进行处理。
在这段代码中,如果 ids 不为空,会进行一些条件判断和操作。首先判断 primaryKey 是否等于 CommonConstant.DEPART_KEY_ORG_CODE,如果是,则调用 i.in 方法,并将 SysDepart::getOrgCode 和 ids 通过逗号分割后的数组作为参数;如果不是,则调用 i.in 方法,并将 SysDepart::getId 和 ids 通过逗号分割后的数组作为参数。
如果 ids 为空,会进行另一些条件判断和操作。首先判断 parentId 是否为空,如果为空,则调用 i.and 方法,并在其中使用 q->q.isNull(true,SysDepart::getParentId).or().eq(true,SysDepart::getParentId,"") 的条件;如果不为空,则调用 i.eq 方法,并将 parentId 作为参数。
总体来说,这段代码根据一些条件对 LambdaQueryWrapper<SysDepart> 进行了不同的操作,具体的操作逻辑可能需要结合上下文来理解。
相关问题
优化这段代码:List<CompletableFuture<Map<String, Object>>> futureList = new ArrayList<>(); for (PersonBasicVo person : personList) { CompletableFuture<Map<String, Object>> future = CompletableFuture.supplyAsync(() -> { String personNo = person.getPersonNo(); List<ContactsBasic> contactsList = contactsService.list(Wrappers.lambdaQuery(ContactsBasic.class) .eq(ContactsBasic::getPersonNo, personNo) .ne(ContactsBasic::getRelationshipAccount, "") .isNotNull(ContactsBasic::getPersonNo) .like(StrUtil.isNotBlank(phone), ContactsBasic::getRelationshipAccount, phone)); if (CollUtil.isNotEmpty(contactsList)) { Map<String, Object> contactsMap = new HashMap<>(); contactsMap.put("personNo", personNo); contactsMap.put("contactsList", contactsList); return contactsMap; } else { return null; } }, executor); futureList.add(future); } List<Map<String, Object>> contactsMapList = futureList.stream() .map(CompletableFuture::join) .filter(Objects::nonNull) .collect(Collectors.toList());
优化后的代码如下:
```java
List<CompletableFuture<Map<String, Object>>> futureList = personList.stream()
.map(person -> CompletableFuture.supplyAsync(() -> {
String personNo = person.getPersonNo();
List<ContactsBasic> contactsList = contactsService.list(Wrappers.lambdaQuery(ContactsBasic.class)
.eq(ContactsBasic::getPersonNo, personNo)
.ne(ContactsBasic::getRelationshipAccount, "")
.isNotNull(ContactsBasic::getPersonNo)
.like(StrUtil.isNotBlank(phone), ContactsBasic::getRelationshipAccount, phone));
if (CollUtil.isNotEmpty(contactsList)) {
Map<String, Object> contactsMap = new HashMap<>();
contactsMap.put("personNo", personNo);
contactsMap.put("contactsList", contactsList);
return contactsMap;
} else {
return null;
}
}, executor))
.collect(Collectors.toList());
List<Map<String, Object>> contactsMapList = futureList.stream()
.map(CompletableFuture::join)
.filter(Objects::nonNull)
.collect(Collectors.toList());
```
主要优化:
1. 使用流式编程,替换原来的for循环。
2. 使用`stream()`和`collect(Collectors.toList())`方法来收集结果,代替手动添加到列表中。
3. 将lambda表达式内联,使代码更简洁。
帮我优化这段代码 private Map<String, Object> boTOMap(DownloadEpidHfmCaseSevereDeathTableVO tableVO){ this.setSonList(tableVO); List<Map<String, Object>> listMap = CodeToStringUtil.dataObjectProcessing(this.getDataObjectProcessingUtilBO(Arrays.asList(tableVO), true, chinesePattern, kg)); if(CollectionUtils.isNotEmpty(listMap)){ Map<String, Object> objectMap = listMap.get(0); //疫苗 List<EpidHfmCaseSevereDeathTestTableVO> selfTestTableVOS = tableVO.getTestTableVOS().stream().filter(item -> item.getJcdx() == DetectionObjectEnum.SELF).collect(Collectors.toList()); List<EpidHfmCaseSevereDeathTestTableVO> familyMembersTestTableVOS = tableVO.getTestTableVOS().stream().filter(item -> item.getJcdx() == DetectionObjectEnum.FAMILY_MEMBERS).collect(Collectors.toList()); if(CollectionUtils.isNotEmpty(selfTestTableVOS)){ objectMap.put("selfTestTable", this.testTableToMap(selfTestTableVOS)); } if(CollectionUtils.isNotEmpty(familyMembersTestTableVOS)){ objectMap.put("familyMembersTestTable", this.testTableToMap(familyMembersTestTableVOS)); } if(CollectionUtils.isNotEmpty(tableVO.getVaccinateTableVOS())){ objectMap.put("vaccinateTable", this.vaccinateTableToMap(tableVO.getVaccinateTableVOS())); } List<Map<String, Object>> dateList = epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableDateList1(tableVO.getId()); dateList.addAll(epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableDateList2(tableVO.getId(), 7-dateList.size())); if(dateList.size() < 7){ int size = dateList.size(); for (int i = 0; i < (7-size); i++) { Map<String, Object> m = new HashMap<>(); m.put("date", "-"); dateList.add(m); } } objectMap.put("titleList", dateList); if(CollectionUtils.isNotEmpty(tableVO.getComplicationTableVOS())){ List<Map<String, Object>> complicationList = null; Map<ComplicationTypeEnum, List<EpidHfmCaseSevereDeathComplicationTableVO>> listMap1 = tableVO.getComplicationTableVOS().stream().collect(Collectors.groupingBy(EpidHfmCaseSevereDeathComplicationTableVO::getType)); int num = 0; for (int j = 0; j < ComplicationTypeEnum.values().length; j++) { complicationList = new ArrayList<>(); List<Map<String, Object>> bfzmcList = epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableBfzmcList( ComplicationTypeEnum.values()[j], tableVO.getId()); for (Map<String, Object> bfzmc : bfzmcList) { Map<String, Object> complication = new HashMap<>(); complication.put("bfzmc7", bfzmc.get("bfzmc")); for (int i = 0; i < dateList.size(); i++) { num = 0; for (EpidHfmCaseSevereDeathComplicationTableVO complicationTableVO : listMap1.get(ComplicationTypeEnum.values()[j])) { if(complicationTableVO.getDate().equals(dateList.get(i).get("title")) && complicationTableVO.getBfzmc().equals(bfzmc.get("bfzmc"))){ complication.put("bfzmc"+i, StringUtils.isNotBlank(complicationTableVO.getJg()) ? complicationTableVO.getJg() : ""); num = 1; break; } } if(num == 0){ complication.put("bfzmc"+i, ""); } } complicationList.add(complication); } objectMap.put("complicationType"+j, complicationList); } } return objectMap; } return null; }
首先,这段代码缺少注释,不容易理解代码的作用。建议加入注释,增加可读性。
其次,可以将一些重复的代码抽取成方法,提高代码复用性和可维护性。例如:
1. 抽取出获取测试表和疫苗表的方法:
```
private void addTestTableAndVaccinateTable(Map<String, Object> objectMap, DownloadEpidHfmCaseSevereDeathTableVO tableVO) {
List<EpidHfmCaseSevereDeathTestTableVO> selfTestTableVOS = tableVO.getTestTableVOS().stream().filter(item -> item.getJcdx() == DetectionObjectEnum.SELF).collect(Collectors.toList());
List<EpidHfmCaseSevereDeathTestTableVO> familyMembersTestTableVOS = tableVO.getTestTableVOS().stream().filter(item -> item.getJcdx() == DetectionObjectEnum.FAMILY_MEMBERS).collect(Collectors.toList());
if(CollectionUtils.isNotEmpty(selfTestTableVOS)){
objectMap.put("selfTestTable", this.testTableToMap(selfTestTableVOS));
}
if(CollectionUtils.isNotEmpty(familyMembersTestTableVOS)){
objectMap.put("familyMembersTestTable", this.testTableToMap(familyMembersTestTableVOS));
}
if(CollectionUtils.isNotEmpty(tableVO.getVaccinateTableVOS())){
objectMap.put("vaccinateTable", this.vaccinateTableToMap(tableVO.getVaccinateTableVOS()));
}
}
private Map<String, Object> testTableToMap(List<EpidHfmCaseSevereDeathTestTableVO> testTableVOS) {
// TODO: 实现将测试表转为 Map 的逻辑
}
private Map<String, Object> vaccinateTableToMap(List<EpidHfmCaseSevereDeathVaccinateTableVO> vaccinateTableVOS) {
// TODO: 实现将疫苗表转为 Map 的逻辑
}
```
2. 抽取出获取并补全并发症表格的方法:
```
private void addComplicationTable(Map<String, Object> objectMap, DownloadEpidHfmCaseSevereDeathTableVO tableVO, List<Map<String, Object>> dateList) {
if(CollectionUtils.isNotEmpty(tableVO.getComplicationTableVOS())){
List<Map<String, Object>> complicationList = null;
Map<ComplicationTypeEnum, List<EpidHfmCaseSevereDeathComplicationTableVO>> listMap1 = tableVO.getComplicationTableVOS().stream().collect(Collectors.groupingBy(EpidHfmCaseSevereDeathComplicationTableVO::getType));
int num = 0;
for (int j = 0; j < ComplicationTypeEnum.values().length; j++) {
complicationList = new ArrayList<>();
List<Map<String, Object>> bfzmcList = epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableBfzmcList(ComplicationTypeEnum.values()[j], tableVO.getId());
for (Map<String, Object> bfzmc : bfzmcList) {
Map<String, Object> complication = new HashMap<>();
complication.put("bfzmc7", bfzmc.get("bfzmc"));
for (int i = 0; i < dateList.size(); i++) {
num = 0;
for (EpidHfmCaseSevereDeathComplicationTableVO complicationTableVO : listMap1.get(ComplicationTypeEnum.values()[j])) {
if(complicationTableVO.getDate().equals(dateList.get(i).get("title")) && complicationTableVO.getBfzmc().equals(bfzmc.get("bfzmc"))){
complication.put("bfzmc"+i, StringUtils.isNotBlank(complicationTableVO.getJg()) ? complicationTableVO.getJg() : "");
num = 1;
break;
}
}
if(num == 0){
complication.put("bfzmc"+i, "");
}
}
complicationList.add(complication);
}
objectMap.put("complicationType"+j, complicationList);
}
}
}
```
这样,原来的代码可以简化为:
```
private Map<String, Object> boTOMap(DownloadEpidHfmCaseSevereDeathTableVO tableVO) {
this.setSonList(tableVO);
List<Map<String, Object>> listMap = CodeToStringUtil.dataObjectProcessing(this.getDataObjectProcessingUtilBO(Arrays.asList(tableVO), true, chinesePattern, kg));
if(CollectionUtils.isNotEmpty(listMap)){
Map<String, Object> objectMap = listMap.get(0);
addTestTableAndVaccinateTable(objectMap, tableVO);
List<Map<String, Object>> dateList = epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableDateList1(tableVO.getId());
dateList.addAll(epidHfmCaseSevereDeathComplicationTableDao.getComplicationTableDateList2(tableVO.getId(), 7-dateList.size()));
if(dateList.size() < 7){
int size = dateList.size();
for (int i = 0; i < (7-size); i++) {
Map<String, Object> m = new HashMap<>();
m.put("date", "-");
dateList.add(m);
}
}
objectMap.put("titleList", dateList);
addComplicationTable(objectMap, tableVO, dateList);
return objectMap;
}
return null;
}
```
相关推荐
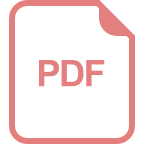
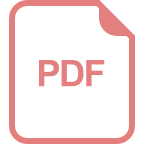
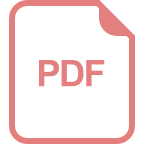













