stm32串口触摸屏代码、
时间: 2023-10-23 08:10:58 浏览: 156
以下是一个基于STM32的串口触摸屏代码示例:
```c
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "stm32f10x.h"
#define USART1_DR_Base 0x40013804
#define USART_RX_BUF_SIZE 64
uint8_t USART_RX_BUF[USART_RX_BUF_SIZE]; //串口接收缓冲区
uint8_t USART_RX_STA = 0; //接收状态标记
//串口1初始化
void USART1_Init(u32 bound)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE); //使能USART1,GPIOA时钟
//USART1_TX PA.9
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; //PA.9
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; //复用推挽输出
GPIO_Init(GPIOA, &GPIO_InitStructure); //初始化PA9
//USART1_RX PA.10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; //PA10
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; //浮空输入
GPIO_Init(GPIOA, &GPIO_InitStructure); //初始化PA10
//Usart1 NVIC 配置
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; //USART1中断
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 3; //抢占优先级3
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3; //子优先级3
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ通道使能
NVIC_Init(&NVIC_InitStructure); //初始化NVIC
//USART 初始化设置
USART_InitStructure.USART_BaudRate = bound;//串口波特率
USART_InitStructure.USART_WordLength = USART_WordLength_8b;//字长为8位数据格式
USART_InitStructure.USART_StopBits = USART_StopBits_1;//一个停止位
USART_InitStructure.USART_Parity = USART_Parity_No;//无奇偶校验位
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;//无硬件数据流控制
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; //收发模式
USART_Init(USART1, &USART_InitStructure); //初始化串口1
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);//开启串口接受中断
USART_Cmd(USART1, ENABLE); //使能串口1
}
//串口1中断服务程序
void USART1_IRQHandler(void)
{
uint8_t Res;
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) //接收中断
{
Res = USART_ReceiveData(USART1);//(USART1->DR); //读取接收到的数据
if ((USART_RX_STA & 0x8000) == 0) //接收未完成
{
if (USART_RX_STA & 0x4000) //接收到了0x0d
{
if (Res != 0x0a)USART_RX_STA = 0; //接收错误,重新开始
else USART_RX_STA |= 0x8000; //接收完成了
}
else //还没收到0X0D
{
if (Res == 0x0d)USART_RX_STA |= 0x4000;
else
{
USART_RX_BUF[USART_RX_STA & 0X3FFF] = Res ;
USART_RX_STA++;
if (USART_RX_STA > (USART_RX_BUF_SIZE - 1))USART_RX_STA = 0;//接收数据错误,重新开始接收
}
}
}
}
}
//向串口1发送一个字节数据
void USART1_SendByte(uint8_t txdata)
{
while((USART1->SR&0X40)==0);//等待上一次发送完毕
USART_SendData(USART1, txdata);
}
//向串口1发送字符串
void USART1_SendString(char* str)
{
uint16_t len = strlen(str);
for (uint16_t i = 0; i < len; i++)
{
USART1_SendByte(*(str + i));
}
}
//发送触摸屏指令
void TouchScreen_SendCommand(uint8_t *cmd, uint16_t cmdlen)
{
USART1_SendByte(0x55); //帧头
USART1_SendByte(0xff); //帧头
USART1_SendByte(0xff); //帧头
for (uint16_t i = 0; i < cmdlen; i++) //发送指令
{
USART1_SendByte(*(cmd + i));
}
USART1_SendByte(0x00); //校验和
}
//触摸屏初始化
void TouchScreen_Init(void)
{
uint8_t cmd1[6] = {0x00, 0x38, 0x00, 0x00, 0x00, 0x00}; //查询芯片ID指令
uint8_t cmd2[6] = {0x00, 0x20, 0x00, 0x02, 0x0d, 0x4e}; //设置坐标读取模式指令
TouchScreen_SendCommand(cmd1, 6); //查询芯片ID
TouchScreen_SendCommand(cmd2, 6); //设置坐标读取模式
}
//从串口1接收一个字节数据
uint8_t USART1_RecvByte(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
//获取触摸屏坐标
void Get_TouchScreen(uint16_t *x, uint16_t *y)
{
uint8_t cmd[6] = {0x00, 0xd0, 0x00, 0x00, 0x00, 0x00}; //读取坐标指令
uint8_t buf[4] = {0};
TouchScreen_SendCommand(cmd, 6); //发送读取坐标指令
for (uint8_t i = 0; i < 4; i++) //等待接收数据
{
buf[i] = USART1_RecvByte();
}
*x = buf[1] << 8 | buf[0];
*y = buf[3] << 8 | buf[2];
}
int main(void)
{
USART1_Init(115200); //初始化串口1
TouchScreen_Init(); //初始化触摸屏
while (1)
{
uint16_t x = 0, y = 0;
Get_TouchScreen(&x, &y);
printf("x: %d, y: %d\r\n", x, y); //打印坐标到串口
}
}
```
此代码实现了通过STM32的串口1与触摸屏进行通信,读取触摸屏的坐标信息,并通过串口打印输出坐标信息。
阅读全文
相关推荐
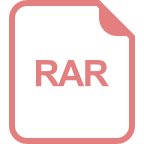
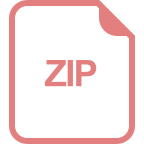
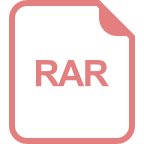
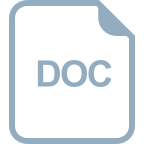

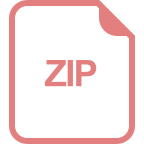
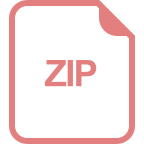
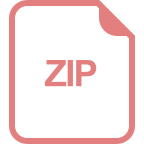
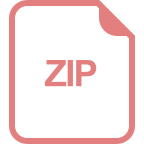
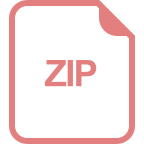
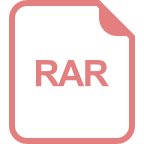
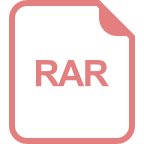
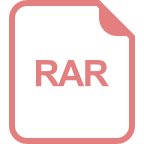
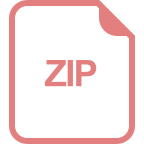
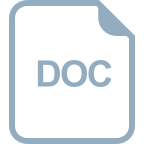