如何使用 R 语言实现矩阵相乘?
时间: 2024-06-18 10:05:03 浏览: 11
使用 R 语言实现矩阵相乘可以通过使用 ` %*%` 运算符来实现,该运算符可以对两个矩阵进行乘法运算。具体操作如下:
假设有两个矩阵 A 和 B,它们的维度分别为 m × n 和 n × p,那么它们的乘积 C 就是一个 m × p 的矩阵。在 R 语言中,可以通过以下代码实现矩阵相乘:
```
# 定义两个矩阵
A <- A %*% B
```
上述代码中,`matrix()` 函数用于定义矩阵,`nrow` 和 `ncol` 参数分别用于指定矩阵的行数和列数。`%*%` 运算符用于对两个矩阵进行乘法运算,结果存储在变量 C 中。
相关问题
R语言 矩阵按行相乘
R语言是一种用于数据分析和统计建模的编程语言。它提供了丰富的数据处理、数据可视化和统计分析的功能。R语言中的矩阵按行相乘可以通过使用`*`运算符来实现。
假设我们有两个矩阵A和B,它们的维度分别为m×n和n×p。要实现矩阵按行相乘,我们可以使用`apply()`函数结合匿名函数来实现。具体步骤如下:
1. 创建两个矩阵A和B:
```R
A <- matrix(c(1, 2, 3, 4), nrow = 2, ncol = 2)
B <- matrix(c(5, 6, 7, 8), nrow = 2, ncol = 2)
```
2. 使用`apply()`函数按行遍历矩阵A,并对每一行进行相应的操作:
```R
result <- apply(A, 1, function(row) {
row * B
})
```
在上述代码中,`apply(A, 1, ...)`表示按行遍历矩阵A,`function(row) { row * B }`表示对每一行进行相应的操作,即将每一行与矩阵B进行相乘。
3. 最后,我们可以通过`result`变量来获取按行相乘后的结果:
```R
result
```
这样,我们就可以得到矩阵按行相乘的结果。
r语言实现JIAJIANCHENGCHU
在R语言中,实现加减乘除的方法如下:
1. 加法:使用"+"运算符进行相加。例如,可以使用表达式a + b来计算a和b的和。
2. 减法:使用"-"运算符进行相减。例如,可以使用表达式a - b来计算a和b的差。
3. 乘法:使用"*"运算符进行相乘。例如,可以使用表达式a * b来计算a和b的积。
4. 除法:使用"/"运算符进行相除。例如,可以使用表达式a / b来计算a除以b的结果。
需要注意的是,R语言中的加减乘除运算符可以用于不同类型的数据,包括数字、向量、矩阵等。同时,R语言也支持其他更高级的数学函数和操作符,如求幂、取余等。
请根据您的具体需求,在R语言中使用适当的运算符和函数来进行加减乘除运算。
相关推荐
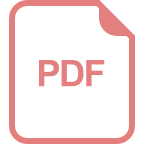
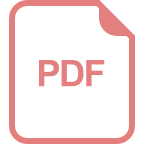












