selenium下拉框
时间: 2023-09-04 17:06:38 浏览: 170
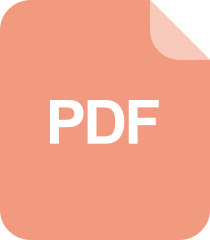
Selenium处理select标签的下拉框

在selenium中操作下拉框,可以使用`Select`类。首先需要导入`from selenium.webdriver.support.ui import Select`。
然后定位到下拉框元素,创建`Select`对象。比如,假设下拉框的id是"fruit",可以这样操作:
```python
from selenium.webdriver.support.ui import Select
select = Select(driver.find_element_by_id("fruit"))
```
之后就可以通过下拉框的值、索引、文本等方式进行选择,例如:
```python
# 根据选项的value属性选择
select.select_by_value("apple")
# 根据选项的索引选择
select.select_by_index(1)
# 根据选项的可见文本选择
select.select_by_visible_text("Banana")
```
如果要获取当前选中的选项,可以使用`first_selected_option`属性获取:
```python
selected_option = select.first_selected_option
```
完整代码示例:
```python
from selenium import webdriver
from selenium.webdriver.support.ui import Select
driver = webdriver.Chrome()
driver.get("https://www.w3schools.com/tags/tryit.asp?filename=tryhtml_select")
# 切换到iframe
iframe = driver.find_element_by_xpath("//iframe[@id='iframeResult']")
driver.switch_to.frame(iframe)
# 选择下拉框
select = Select(driver.find_element_by_id("cars"))
# 选择第二个选项
select.select_by_index(1)
selected_option = select.first_selected_option
print(selected_option.text)
# 选择第三个选项
select.select_by_index(2)
selected_option = select.first_selected_option
print(selected_option.text)
# 返回默认的选项
select.select_by_index(0)
selected_option = select.first_selected_option
print(selected_option.text)
driver.quit()
```
阅读全文
相关推荐
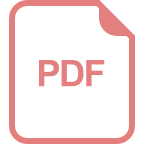
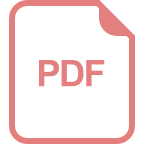














