编写 PHP 页面实现一个留言板,包括留言者的 id(可以是网名),时间, 主题,正文和附件;另外设计一个页面对留言进行列表,以表格的形式展示留言 的简介;当点击一条信息后,显示该留言的详细信息
时间: 2023-11-13 17:05:57 浏览: 39
以下是实现留言板和留言列表的 PHP 代码:
**留言板页面(message_board.php):**
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<form action="submit_message.php" method="post" enctype="multipart/form-data">
<label for="id">留言者ID:</label>
<input type="text" name="id" required><br>
<label for="title">主题:</label>
<input type="text" name="title" required><br>
<label for="content">正文:</label>
<textarea name="content" required></textarea><br>
<label for="attachment">附件:</label>
<input type="file" name="attachment"><br>
<input type="submit" value="提交留言">
</form>
</body>
</html>
```
**留言提交页面(submit_message.php):**
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>提交留言</title>
</head>
<body>
<?php
// 获取留言数据
$id = $_POST['id'];
$title = $_POST['title'];
$content = $_POST['content'];
// 处理附件上传
$attachment = '';
if ($_FILES['attachment']['error'] == UPLOAD_ERR_OK) {
$attachment = $_FILES['attachment']['name'];
move_uploaded_file($_FILES['attachment']['tmp_name'], './attachments/' . $attachment);
}
// 保存留言数据到文件
$message = array(
'id' => $id,
'time' => time(),
'title' => $title,
'content' => $content,
'attachment' => $attachment
);
$messages = array();
if (file_exists('messages.json')) {
$messages = json_decode(file_get_contents('messages.json'), true);
}
array_push($messages, $message);
file_put_contents('messages.json', json_encode($messages));
echo '<p>留言提交成功!</p>';
?>
<a href="message_board.php">返回留言板</a>
</body>
</html>
```
**留言列表页面(message_list.php):**
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>留言列表</title>
</head>
<body>
<h1>留言列表</h1>
<table>
<tr>
<th>ID</th>
<th>时间</th>
<th>主题</th>
<th>附件</th>
</tr>
<?php
// 从文件中读取留言数据
$messages = array();
if (file_exists('messages.json')) {
$messages = json_decode(file_get_contents('messages.json'), true);
}
// 显示留言列表
foreach ($messages as $message) {
echo '<tr>';
echo '<td>' . $message['id'] . '</td>';
echo '<td>' . date('Y-m-d H:i:s', $message['time']) . '</td>';
echo '<td><a href="message_detail.php?index=' . array_search($message, $messages) . '">' . $message['title'] . '</a></td>';
if ($message['attachment'] != '') {
echo '<td><a href="./attachments/' . $message['attachment'] . '">' . $message['attachment'] . '</a></td>';
} else {
echo '<td></td>';
}
echo '</tr>';
}
?>
</table>
</body>
</html>
```
**留言详情页面(message_detail.php):**
```php
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>留言详情</title>
</head>
<body>
<?php
// 获取留言数据
$messages = array();
if (file_exists('messages.json')) {
$messages = json_decode(file_get_contents('messages.json'), true);
}
$index = $_GET['index'];
$message = $messages[$index];
// 显示留言详情
echo '<h1>' . $message['title'] . '</h1>';
echo '<p>ID:' . $message['id'] . '</p>';
echo '<p>时间:' . date('Y-m-d H:i:s', $message['time']) . '</p>';
echo '<p>正文:</p>';
echo '<p>' . $message['content'] . '</p>';
if ($message['attachment'] != '') {
echo '<p>附件:<a href="./attachments/' . $message['attachment'] . '">' . $message['attachment'] . '</a></p>';
}
?>
<a href="message_list.php">返回留言列表</a>
</body>
</html>
```
注意事项:
1. 以上代码中使用了 JSON 格式保存留言数据,需要确保服务器支持 JSON 相关函数;
2. 留言附件的上传和下载需要确保服务器具有相应权限;
3. 以上代码中并未进行安全性和异常处理,需要根据实际情况进行完善。
相关推荐
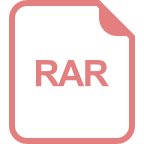
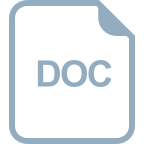














