java完整实现websocket服务器端
时间: 2023-09-25 12:16:28 浏览: 59
以下是一个简单的 Java WebSocket 服务器端的完整实现:
```java
import javax.websocket.*;
import javax.websocket.server.ServerEndpoint;
import java.io.IOException;
import java.util.concurrent.CopyOnWriteArrayList;
@ServerEndpoint("/websocket")
public class WebSocketServer {
private static CopyOnWriteArrayList<Session> sessions = new CopyOnWriteArrayList<>();
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
System.out.println("WebSocket opened: " + session.getId());
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("WebSocket message received: " + message);
broadcast(message);
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
System.out.println("WebSocket closed: " + session.getId());
}
@OnError
public void onError(Throwable throwable) {
System.out.println("WebSocket error: " + throwable.getMessage());
}
private void broadcast(String message) {
for (Session session : sessions) {
try {
session.getBasicRemote().sendText(message);
} catch (IOException e) {
System.out.println("Failed to send message to session: " + session.getId());
sessions.remove(session);
}
}
}
}
```
该实现使用了 Java WebSocket API,并使用了 `@ServerEndpoint` 注解将其声明为 WebSocket 服务器端点。在 `onOpen()` 方法中,会将新连接的 `Session` 对象添加到 `sessions` 列表中。在 `onMessage()` 方法中,会将接收到的消息进行广播,即发送给所有连接的客户端。在 `onClose()` 方法中,会将关闭的 `Session` 对象从 `sessions` 列表中移除。在 `onError()` 方法中,会输出错误信息。`broadcast()` 方法用于将消息发送给所有连接的客户端。
该实现使用了一个 `CopyOnWriteArrayList` 对象来存储所有连接的 `Session` 对象,这个对象是线程安全的,因此在多个线程中对其进行读写是安全的。在 `broadcast()` 方法中,会遍历 `sessions` 列表,并向每个客户端发送消息。如果发送消息失败,则会将该客户端的 `Session` 对象从 `sessions` 列表中移除。
要在 Tomcat 中运行该 WebSocket 服务器端,需要在 `web.xml` 文件中添加以下配置:
```xml
<websocket-endpoint>
<endpoint-class>WebSocketServer</endpoint-class>
</websocket-endpoint>
```
然后,在 Tomcat 中启动应用程序即可。现在,您可以使用任何 WebSocket 客户端连接到该服务器端了。
相关推荐
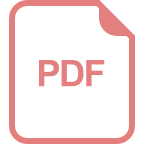
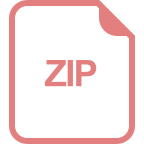
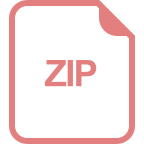














