python文件递归
时间: 2024-01-10 11:50:04 浏览: 21
要在Python中实现文件递归,可以使用os模块的相关函数。下面是一个示例代码,使用递归方式遍历目录并打印所有文件的路径:
```python
import os
def recursive_file_search(directory):
for item in os.listdir(directory):
item_path = os.path.join(directory, item)
if os.path.isfile(item_path):
print(item_path)
elif os.path.isdir(item_path):
recursive_file_search(item_path)
# 调用该函数并传入要遍历的目录路径
recursive_file_search('/path/to/directory')
```
在上面的代码中,`recursive_file_search`函数使用递归方式遍历指定目录。对于每个项目,如果它是文件,则打印其路径;如果是目录,则递归调用`recursive_file_search`函数以进一步遍历该目录。你需要将`/path/to/directory`替换为你要遍历的实际目录路径。
需要注意的是,使用递归方式遍历目录时要小心避免无限递归。确保目录结构是正确的,且没有循环引用等问题。此外,对于大型目录结构,递归可能会导致性能问题,可以考虑使用迭代或其他优化方法来处理。
相关问题
python递归解压缩文件
可以使用Python的zipfile模块来解压缩文件,以下是递归解压缩文件的代码:
```python
import os
import zipfile
def unzip_file(path, dest):
with zipfile.ZipFile(path, 'r') as zip_ref:
zip_ref.extractall(dest)
for root, dirs, files in os.walk(dest):
for file in files:
if file.endswith('.zip'):
file_path = os.path.join(root, file)
unzip_file(file_path, dest)
```
其中,path是要解压缩的文件路径,dest是解压缩后的目标文件夹路径。这段代码会递归地解压缩所有嵌套的zip文件。
使用python递归解压zip文件
可以使用Python内置的zipfile模块来解压zip文件,以下是一个递归解压zip文件的示例代码:
```python
import os
import zipfile
def extract_all_zip_files(path):
"""
递归解压指定目录下的所有zip文件
"""
for dirpath, dirnames, filenames in os.walk(path):
for filename in filenames:
if filename.endswith('.zip'):
zip_file_path = os.path.join(dirpath, filename)
with zipfile.ZipFile(zip_file_path, 'r') as zip_file:
zip_file.extractall(dirpath)
os.remove(zip_file_path)
for dirname in dirnames:
extract_all_zip_files(os.path.join(dirpath, dirname))
```
将需要解压的zip文件所在的顶级目录路径传入该函数即可递归解压该目录下的所有zip文件。函数会自动删除解压后的zip文件。
相关推荐
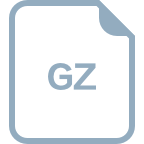












