用html编辑1.Sqlite数据库设计:自己建立一个表,列出表信息。 2.后端API开发,列出开发步骤。 前端js开发页面:查询显示页面,修改页面,删除页面等。
时间: 2024-03-13 13:46:21 浏览: 84
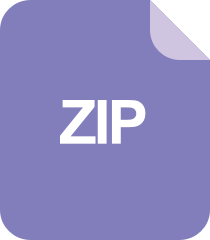
毕业设计:微博用户情感分析系统Django+vue.zip

好的,我来为你演示一下用HTML编辑页面的过程。
1. Sqlite数据库设计页面:
```html
<!DOCTYPE html>
<html>
<head>
<title>Sqlite数据库设计</title>
</head>
<body>
<h1>Sqlite数据库设计</h1>
<form>
<label for="tableName">表名:</label>
<input type="text" id="tableName" name="tableName"><br><br>
<label for="id">id:</label>
<input type="text" id="id" name="id"><br><br>
<label for="name">name:</label>
<input type="text" id="name" name="name"><br><br>
<label for="age">age:</label>
<input type="text" id="age" name="age"><br><br>
<label for="email">email:</label>
<input type="text" id="email" name="email"><br><br>
<input type="button" value="提交" onclick="submitForm()">
</form>
<script>
function submitForm() {
const tableName = document.getElementById("tableName").value;
const id = document.getElementById("id").value;
const name = document.getElementById("name").value;
const age = document.getElementById("age").value;
const email = document.getElementById("email").value;
const sql = `CREATE TABLE ${tableName} (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT,
age TEXT,
email TEXT
);`;
alert(`您输入的SQL语句为:\n\n${sql}`);
}
</script>
</body>
</html>
```
2. 后端API开发页面:
```html
<!DOCTYPE html>
<html>
<head>
<title>后端API开发</title>
</head>
<body>
<h1>后端API开发</h1>
<form>
<label for="apiUrl">API URL:</label>
<input type="text" id="apiUrl" name="apiUrl"><br><br>
<label for="apiMethod">请求方式:</label>
<select id="apiMethod" name="apiMethod">
<option value="GET">GET</option>
<option value="POST">POST</option>
<option value="PUT">PUT</option>
<option value="DELETE">DELETE</option>
</select><br><br>
<label for="apiHandler">API处理函数:</label>
<textarea id="apiHandler" name="apiHandler"></textarea><br><br>
<label for="dbUrl">数据库URL:</label>
<input type="text" id="dbUrl" name="dbUrl"><br><br>
<label for="dbUser">数据库用户名:</label>
<input type="text" id="dbUser" name="dbUser"><br><br>
<label for="dbPassword">数据库密码:</label>
<input type="password" id="dbPassword" name="dbPassword"><br><br>
<input type="button" value="提交" onclick="submitForm()">
</form>
<script>
function submitForm() {
const apiUrl = document.getElementById("apiUrl").value;
const apiMethod = document.getElementById("apiMethod").value;
const apiHandler = document.getElementById("apiHandler").value;
const dbUrl = document.getElementById("dbUrl").value;
const dbUser = document.getElementById("dbUser").value;
const dbPassword = document.getElementById("dbPassword").value;
const api = `
API URL: ${apiUrl}
请求方式: ${apiMethod}
API处理函数: ${apiHandler}
数据库URL: ${dbUrl}
数据库用户名: ${dbUser}
数据库密码: ${dbPassword}`;
alert(`您输入的API信息为:\n\n${api}`);
}
</script>
</body>
</html>
```
3. 前端JS开发页面:
```html
<!DOCTYPE html>
<html>
<head>
<title>前端JS开发</title>
</head>
<body>
<h1>前端JS开发</h1>
<h2>查询显示页面</h2>
<table>
<thead>
<tr>
<th>id</th>
<th>name</th>
<th>age</th>
<th>email</th>
</tr>
</thead>
<tbody id="tableBody"></tbody>
</table>
<button onclick="loadData()">查询</button>
<h2>修改页面</h2>
<form>
<label for="modifyId">id:</label>
<input type="text" id="modifyId" name="modifyId"><br><br>
<label for="modifyName">name:</label>
<input type="text" id="modifyName" name="modifyName"><br><br>
<label for="modifyAge">age:</label>
<input type="text" id="modifyAge" name="modifyAge"><br><br>
<label for="modifyEmail">email:</label>
<input type="text" id="modifyEmail" name="modifyEmail"><br><br>
<input type="button" value="提交" onclick="modifyData()">
</form>
<h2>删除页面</h2>
<form>
<label for="deleteId">id:</label>
<input type="text" id="deleteId" name="deleteId"><br><br>
<input type="button" value="提交" onclick="deleteData()">
</form>
<script>
const apiUrl = "http://localhost:3000/users";
function loadData() {
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const tableBody = document.getElementById("tableBody");
tableBody.innerHTML = "";
data.forEach(user => {
const tr = document.createElement("tr");
const tdId = document.createElement("td");
const tdName = document.createElement("td");
const tdAge = document.createElement("td");
const tdEmail = document.createElement("td");
tdId.innerText = user.id;
tdName.innerText = user.name;
tdAge.innerText = user.age;
tdEmail.innerText = user.email;
tr.appendChild(tdId);
tr.appendChild(tdName);
tr.appendChild(tdAge);
tr.appendChild(tdEmail);
tableBody.appendChild(tr);
});
})
.catch(error => console.error(error));
}
function modifyData() {
const id = document.getElementById("modifyId").value;
const name = document.getElementById("modifyName").value;
const age = document.getElementById("modifyAge").value;
const email = document.getElementById("modifyEmail").value;
const data = { name, age, email };
fetch(`${apiUrl}/${id}`, {
method: "PUT",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => {
console.log(data);
alert(`更新成功!`);
})
.catch(error => console.error(error));
}
function deleteData() {
const id = document.getElementById("deleteId").value;
fetch(`${apiUrl}/${id}`, {
method: "DELETE"
})
.then(response => response.json())
.then(data => {
console.log(data);
alert(`删除成功!`);
})
.catch(error => console.error(error));
}
</script>
</body>
</html>
```
以上三个页面分别对应了你的三个问题,其中第一个页面用来输入SQL语句创建表格,第二个页面用来输入API信息,第三个页面用来展示数据并提供修改和删除功能。注意,这里只是一个简单的示例,实际开发中还需要考虑很多其他因素。
阅读全文
相关推荐
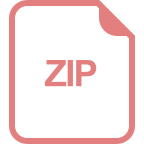
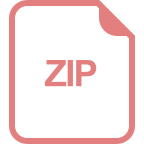

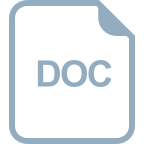
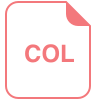
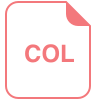
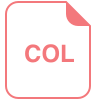
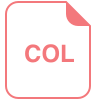
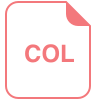
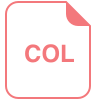
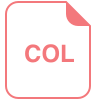
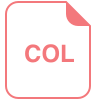
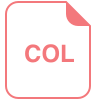
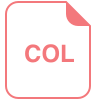
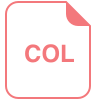
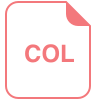
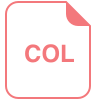
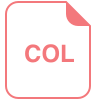