如何利用lstm模型提取一段时序的特征,与其他两个数组一起作为mlp模型的输入层,每个数组为一列有三个数据
时间: 2023-06-24 17:07:48 浏览: 40
可以按照以下步骤进行:
1. 将需要提取特征的时序数据分成多个时间步,每个时间步都是一个三维的向量。
2. 使用LSTM模型对每个时间步的向量进行特征提取,得到一个固定长度的向量作为输入的一维特征。
3. 将得到的一维特征与另外两个数组拼接成一个二维矩阵,作为MLP模型的输入层。
4. 使用MLP模型进行训练和预测。
以下是一个示例代码:
```python
from keras.models import Sequential
from keras.layers import LSTM, Dense
# 根据需要提取特征的时序数据,将其分成多个时间步,每个时间步都是一个三维的向量
time_steps = 10
input_dim = 3
data = [[i+j for i in range(input_dim)] for j in range(100)]
X = []
Y = []
for i in range(len(data)-time_steps):
X.append(data[i:i+time_steps])
Y.append(data[i+time_steps])
# 使用LSTM模型对每个时间步的向量进行特征提取,得到一个固定长度的向量作为输入的一维特征
lstm_model = Sequential()
lstm_model.add(LSTM(32, input_shape=(time_steps, input_dim)))
lstm_model.add(Dense(16, activation='relu'))
lstm_model.add(Dense(8, activation='relu'))
lstm_model.add(Dense(4, activation='relu'))
lstm_model.add(Dense(1))
lstm_model.compile(optimizer='adam', loss='mse')
X_lstm = lstm_model.predict(X)
# 将得到的一维特征与另外两个数组拼接成一个二维矩阵,作为MLP模型的输入层
import numpy as np
X_mlp = np.concatenate([X_lstm, np.random.rand(len(X_lstm), 2), np.random.rand(len(X_lstm), 2)], axis=1)
# 使用MLP模型进行训练和预测
mlp_model = Sequential()
mlp_model.add(Dense(32, input_dim=X_mlp.shape[1], activation='relu'))
mlp_model.add(Dense(16, activation='relu'))
mlp_model.add(Dense(8, activation='relu'))
mlp_model.add(Dense(1))
mlp_model.compile(optimizer='adam', loss='mse')
mlp_model.fit(X_mlp, Y, epochs=100, batch_size=32)
# 预测新数据
new_data = np.random.rand(1, time_steps, input_dim)
new_lstm_feature = lstm_model.predict(new_data)
new_mlp_input = np.concatenate([new_lstm_feature, np.random.rand(1, 2), np.random.rand(1, 2)], axis=1)
mlp_model.predict(new_mlp_input)
```
相关推荐
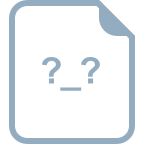
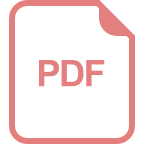
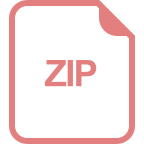










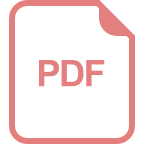
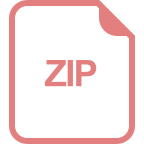
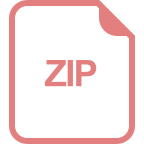
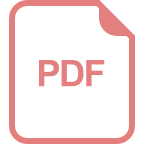