编写程序,从一文本文件中读取若干行,实现将重复的单词存入一个 Set 对象中,并不重复的单词存入另一个 Set 对象中。
时间: 2024-11-25 10:19:15 浏览: 8
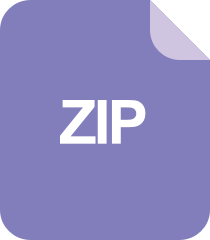
CodingProblems:从 O(n) 中的大字符串中选择最常出现的前 k 个单词
在Java中,你可以使用BufferedReader来逐行读取文本文件,然后使用HashSet(用于存储唯一元素)和TreeSet(保证元素有序且无重复)来分别处理重复和不重复的单词。以下是一个简单的示例代码:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashSet;
import java.util.Set;
import java.util.TreeSet;
public class WordFilter {
public static void main(String[] args) {
String filePath = "path_to_your_file.txt"; // 替换为你的文件路径
HashSet<String> duplicates = new HashSet<>();
TreeSet<String> uniqueWords = new TreeSet<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = reader.readLine()) != null) {
String[] words = line.split("\\s+"); // 分割按空格分隔的单词
for (String word : words) {
if (!duplicates.add(word)) { // 如果word已经在duplicates集合中,则表示重复
System.out.println("Duplicate word found: " + word);
} else {
uniqueWords.add(word); // 将不重复的单词添加到uniqueWords中
}
}
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
System.out.println("\nUnique words:");
for (String word : uniqueWords) {
System.out.println(word);
}
}
}
```
在这个例子中,我们首先创建了一个HashSet(`duplicates`)来存储重复的单词,由于HashSet不允许有重复元素,所以当试图添加一个已经存在的单词时,add方法返回false,我们可以判断并打印出来。
同时,我们将每个非重复单词添加到TreeSet(`uniqueWords`),TreeSet自动保持元素的排序,而且不允许有重复。
阅读全文
相关推荐
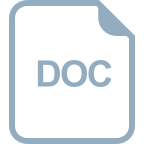
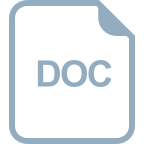



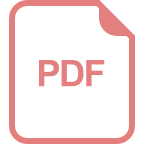











