c实现aes-cmac算法
时间: 2023-09-17 12:03:25 浏览: 703
AES-CMAC是一种使用AES算法实现的消息认证码(MAC)算法。以下是一个用C语言实现的AES-CMAC算法的示例代码:
```c
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
void XOR(unsigned char *a, unsigned char *b, unsigned char *out, int len) {
for(int i = 0; i < len; i++) {
out[i] = a[i] ^ b[i];
}
}
void double_128bit(unsigned char *input, unsigned char *output) {
unsigned char carry = 0x00;
for(int i = 15; i >= 0; i--) {
output[i] = input[i] << 1;
output[i] |= carry;
carry = (input[i] & 0x80) ? 0x01 : 0x00;
}
if((input[0] & 0x80) != 0) {
output[15] ^= 0x87;
}
}
void aes_cmac(unsigned char *key, unsigned char *msg, int msg_len, unsigned char *mac) {
AES_KEY aes_key;
unsigned char L[16], K1[16], K2[16];
unsigned char subkey[16];
unsigned char padded_msg[16];
memset(L, 0x00, sizeof(L));
memset(K1, 0x00, sizeof(K1));
memset(K2, 0x00, sizeof(K2));
aes_set_encrypt_key(key, 128, &aes_key);
aes_encrypt(&aes_key, L, K1);
double_128bit(K1, K2);
int num_blocks = (msg_len + 15) / 16;
int last_block_len = msg_len % 16;
if(last_block_len == 0) {
memcpy(padded_msg, &msg[(num_blocks - 1) * 16], 16);
XOR(padded_msg, K1, subkey, 16);
} else {
memcpy(padded_msg, &msg[(num_blocks - 1) * 16], last_block_len);
padded_msg[last_block_len] = 0x80;
memset(&padded_msg[last_block_len + 1], 0x00, 15 - last_block_len);
XOR(padded_msg, K2, subkey, 16);
}
for(int i = 0; i < num_blocks - 1; i++) {
XOR(subkey, &msg[i * 16], subkey, 16);
aes_encrypt(&aes_key, subkey, subkey);
}
XOR(subkey, padded_msg, mac, 16);
aes_encrypt(&aes_key, mac, mac);
}
int main() {
unsigned char key[16] = {0x2b, 0x7e, 0x15, 0x16, 0x28, 0xae, 0xd2, 0xa6, 0xab, 0xf7, 0x15, 0x88, 0x09, 0xcf, 0x4f, 0x3c};
unsigned char msg[32] = {0x6b, 0xc1, 0xbe, 0xe2, 0x2e, 0x40, 0x9f, 0x96, 0xe9, 0x3d, 0x7e, 0x11, 0x73, 0x93, 0x17, 0x2a, 0xae, 0x2d, 0x8a, 0x57, 0x1e, 0x03, 0xac, 0x9c, 0x9e, 0xb7, 0x6f, 0xac, 0x45, 0xaf, 0x8e, 0x51, 0x30};
unsigned char mac[16];
aes_cmac(key, msg, sizeof(msg), mac);
printf("MAC: ");
for(int i = 0; i < 16; i++) {
printf("%02x", mac[i]);
}
printf("\n");
return 0;
}
```
这是一个简单的AES-CMAC算法的实现。它使用了OpenSSL库来实现AES算法。```aes_cmac```函数接受一个128位的密钥、要计算MAC的消息和消息的长度作为输入,并输出一个128位的MAC。
你可以自行替换```key```和```msg```变量的值以测试该代码的功能。最终计算得到的MAC将会输出到控制台。
需要注意的是,这只是一个简单示例,用于演示如何实现AES-CMAC算法。在实际使用中,你应该使用经过审计和验证的加密库来保护你的数据。
阅读全文
相关推荐
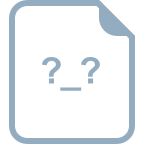

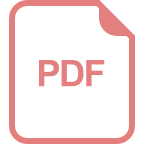
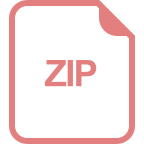
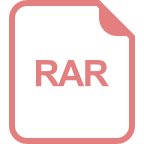
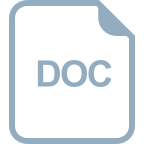







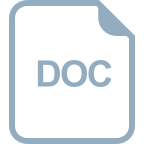

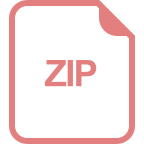