银行家算法java实验
时间: 2024-01-04 18:20:42 浏览: 34
以下是一个使用Java实现银行家算法的示例:
```java
import java.util.Arrays;
public class BankerAlgorithm {
private int[][] max; // 最大需求矩阵
private int[][] allocation; // 已分配矩阵
private int[][] need; // 需求矩阵
private int[] available; // 可利用资源向量
private int[] safeSequence; // 安全序列
public BankerAlgorithm(int[][] max, int[][] allocation, int[] available) {
this.max = max;
this.allocation = allocation;
this.available = available;
int m = max.length; // 进程数
int n = max[0].length; // 资源种类数
need = new int[m][n];
safeSequence = new int[m];
// 计算需求矩阵
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
}
public boolean isSafe() {
int m = max.length; // 进程数
int n = max[0].length; // 资源种类数
boolean[] finish = new boolean[m]; // 完成标记数组
int[] work = Arrays.copyOf(available, n); // 可利用资源向量的副本
int count = 0; // 完成进程数
while (count < m) {
boolean found = false;
for (int i = 0; i < m; i++) {
if (!finish[i] && isNeedLessOrEqual(work, need[i])) {
// 分配资源
for (int j = 0; j < n; j++) {
work[j] += allocation[i][j];
}
safeSequence[count] = i;
finish[i] = true;
count++;
found = true;
}
}
if (!found) {
return false; // 无法找到安全序列
}
}
return true; // 找到安全序列
}
private boolean isNeedLessOrEqual(int[] work, int[] need) {
for (int i = 0; i < work.length; i++) {
if (work[i] < need[i]) {
return false;
}
}
return true;
}
public int[] getSafeSequence() {
return safeSequence;
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
int[][] max = {
{7, 5, 3},
{3, 2, 2},
{9, 0, 2},
{2, 2, 2},
{4, 3, 3}
};
int[][] allocation = {
{0, 1, 0},
{2, 0, 0},
{3, 0, 2},
{2, 1, 1},
{0, 0, 2}
};
int[] available = {3, 3, 2};
BankerAlgorithm bankerAlgorithm = new BankerAlgorithm(max, allocation, available);
if (bankerAlgorithm.isSafe()) {
int[] safeSequence = bankerAlgorithm.getSafeSequence();
System.out.println("安全序列: " + Arrays.toString(safeSequence));
} else {
System.out.println("无法找到安全序列");
}
}
}
```
相关推荐
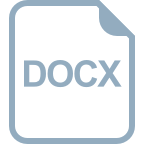
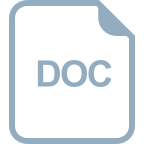
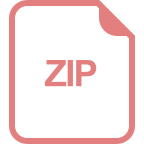















